Listening for KeyPress events with DataWedge
Please be aware that the following article only applies to our devices running the SD660 chipset and some other select devices running other chipsets. If you have DataWedge 7.3 and do not see the Keyevent options page in DataWedge as described then unfortunately this feature is not available on your device.
The recently released DataWedge 7.3 quietly introduced a new feature that customers have been requesting for a long time now, the ability to send data as key events. What this means in practice is an application can now listen for keypress events whereas previously the application could only scan into text fields using the keystroke plugin.
There are a number of advantages to this approach:
- Any web application can now receive barcode scans by listening for key press events on the DOM without having to worry about having the cursor in the correct input field or advancing to the next field after the barcode is scanned.
- Applications written for HID based external scanners can be run on Zebra Android devices without having to modify the application at all.
- Web applications can be run entirely within the Browser without having to worry about external frameworks or native dependencies
- Future compatibility - this is a very simple solution and you are not tying yourself to the hardware manufacturer.
Please see the following GitHub project for sample code.
Within your DataWedge profile navigate to the Keystroke output section, then Key event options to see the above options under DW 7.3.
Option: "Send Characters as Events"
Send Characters as Events is the critical setting for receiving data as keypress events, set it to true to receive any standard text character as a keypress (there is an ASCII table at the bottom of this post for reference) - everything between space and tilde (~).
When enabled, you can say something like the following in your JavaScript:
Sample JavaScript
document.addEventListener('keypress', keypressHandler); function keypressHandler(e) { const keypressoutput = document.getElementById('pressed_keys'); if (e.keyCode == 13) // Enter key from DataWedge keypressoutput.innerHTML += "
"; else keypressoutput.innerHTML += e.key; }
For each scanned barcode the text is delivered to the application as key presses and the above example will appended a "
" whenever it sees the 'Enter' character. The code will appear as follows in Chrome:
The sample page is within this GitHub project and you will find some example barcodes lower down. Yes, I know it does not look very pretty
Determining the end of barcodes
Obviously all this page is doing is listening for keystrokes so the application needs to determine where one barcode finishes and the next one begins. There are a few popular ways to achieve this:
- Where the barcodes are all a fixed length, you can listen for a specific number of characters.
- Where barcodes end in a particular character, you can break on that character (the sample app uses this approach)
- Using DataWedge's Data Formatting you can append a character to the end of the barcode automatically which is the approach I would personally recommend.
- Timing: Humans can only scan so fast so whenever a long enough pause between characters is detected, assume the barcode is complete.
Options: "Send Enter as String" / "Send Tab as String"
These options are not needed by a solution listening for keypress events within the app (or on the DOM for web apps); they still exist for compatibility with applications that scan into text fields, tabbing between those text fields and entering (submitting) the form that contains those text fields.
Option: Send Control Characters as Events
Do NOT assume (as I did) that e.g. sending ASCII code decimal 7 will result in the web page receiving the BELL character. The control characters that this option refers to are defined fully in the "ASCII Control Character Table" present in the official documentation.
For example, ASCII code decimal 7 actually refers to the code equivalent of CTRL+G (decimal 71).
By way of a demonstration, if we enable this option and send a barcode containing the ASCII code decimal 7 we can look at how this event is received by the application:
The barcode used for this is given at the bottom of this document. Note that the ASCII keycode 71 is received in the application for both KEYUP and KEYDOWN but NOT for KEYPRESS, this is expected behaviour since Android will not generate a KEYPRESS event for ASCII code 71. You can observe the same behaviour by loading the test page in a desktop browser and pressing CTRL+G, where you will also receive a keycode 71.
Things to Bear in Mind
- Android will not generate a keypress event for every character, as was noted above you will not get keypresses for many of the control keys (which also includes TAB - ASCII decimal 9).
- Vysor will interfere with the operation of the DataWedge keystroke output plugin on the device as it installs its own keyboard. Personally I find it easier to uninstall Vysor when I am not actively using it.
- DataWedge seems to return ASCII code 13 for the enter key regardless of how the barcode was encoded (with \n or \r). This could be an artifact of how I created the barcodes, I am not sure but I have included both below.
Barcodes
Use the following barcodes to test the functionality of the test page in the GitHub project.
QR Barcode: 1234567890
The above barcode is a standard barcode without any special characters appended to the end.
QR Barcode: 1234567890\n
The above barcode has the ASCII character with decimal 10 (LF) appended to the end of it.
QR Barcode: 1234567890\r
The above barcode has the ASCII character with decimal 13 (CR) appended to the end of it.
QR Barcode: 1234567890\7
The above barcode has the ASCII character with decimal 7 (BELL) appended to the end of it.
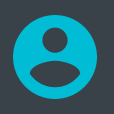
Darryn Campbell
2 Replies
This example really helped us capture barcodes in our web application running under Chrome on a MC3300x. However, we have run into an anomaly that can be reproduced by adding an alert(...) to the above example as shown below:
function keypressHandler(e) {
const keypressoutput = document.getElementById('pressed_keys');
if (e.keyCode == 13) { // Enter key from DataWedge
keypressoutput.innerHTML += "";
alert('hello');
} else {
keypressoutput.innerHTML += e.key;
}
}
When you run a web page with the above in Chrome on a Windows PC, you can dismiss the alert, and any characters you type subsequently show on the web page. When you run the same web page in Chrome on the MC3300x, any characters you type after dismissing the alert do *not* show on the web page until you tap on the MC3300x screen.
Ideas and/or suggestions for a workaround on the MC3300x are welcome. We don't want to have to tap the screen to be able to continue receiving barcode scans. Thanks!
Thank you for the useful content, the article serves as a guide for implementing the new feature in DataWedge 7.3 for barcode scanning applications, especially focusing on web strands applications. It provides practical examples and recommendations for handling barcode data effectively.