Porting your PocketBrowser Application to RhoElements
This blog describes how you would go about porting an existing PocketBrowser application into RhoElements for Motorola devices and how to do so in a high performing fashion.
What is PocketBrowser?
Motorola PocketBrowser is the precursor to RhoElements and RhoMobile Suite within Motorola. It allows developers to write HTML based applications across a range of Motorola Solutions' devices to access the device capabilities of that device such as Scanning and Magnetic Stripe Card reading. Introduced 10 years ago the software continues to be supported in its current form on Motorola's latest Windows devices.
In order to access the device capabilities using PocketBrowser developers use Tags on their HTML pages which expose the underlying hardware. The syntax provided to these meta tags is known as EMML 1.0 (http://docs.rhomobile.com/rhoelements/EMMLOverview#emml-in-its-original-form-emml-10) and it was primarily designed as a declarative rather than an interactive user experience.
Whilst EMML 1.1 was introduced in PocketBrowser 3.0 this blog will assume that all PocketBrowser code is written in EMML1.0 syntax, code already written in EMML1.1 does not require conversion.
The underlying rendering engine of PocketBrowser is Internet Explorer, as shipped on the device and therefore the functionality of the rendering engine (eg. support for CSS) is dependant on the device running your application. In order to access the EMML tags and device capabilities from JavaScript a number of ActiveX objects can be instantiated, the most common of which is 'Generic' and the code below shows how you would enable the scanner using the ActiveX object:
var myObj = new ActiveXObject("PocketBrowser.Generic");
myObj.invokeMETAFunction('Scanner','enable');
Why would I want to port to RhoElements?
RhoElements can be viewed as the latest incarnation of PocketBrowser; whilst PocketBrowser will continue to be maintained for our Enterprise customers in their current device deployments customers are advised to move to RhoElements to take advantage of the additional features on offer.
- Support for the latest Motorola devices. RhoElements will always be ahead of the curve in supporting the latest Motorola Windows products as they enter the market, we are already working on supporting devices which have yet to enter the market.
- There are no plans to support PocketBrowser on any current or future Motorola Android devices, such as the ET1.
- PocketBrowser will not run on the full suite of consumer devices supported by RhoElements, such as iPhone, BlackBerry, consumer Android and Windows
- PocketBrowser only allows for the creation of 'Hybrid' applications, that is applications written in HTML / JavaScript and take advantage of device capabilities. RhoElements offers a plethora of additional development opportunities e.g. support for the MVC pattern, native application development, an Eclipse based IDE, a device simulator, full integration with 'RhoConect' providing seemless syncing with the backend and so on.
- RhoElements has more functional APIs for scanning, image capture and more
- RhoElements has APIs to hardware not exposed through PocketBrowser such as device sensors and NFC.
- RhoElements offers an HTML5 compliant rendering engine allowing you to create beautiful applications, in stark contrast with the Internet Explorer based rendering engine in PocketBrowser.
Native Applications vs. the Shared Runtime
With all this talk of native applications, backend databases, synchronization and IDEs it's difficult to understand how PocketBrowser and RhoElements can be considered similar. To make the transition easier for both existing MPB customers and customers who just want a 'hybrid' application solution we have provided the 'Shared Runtime'. The Shared Runtime can be considered a shell, a cab file (or apk) which can be installed on Windows Mobile / CE / Android devices including the full range of supported Motorola Solutions' devices. Pointing the shared runtime at your HTML start page will launch your application in the HTML5 rendering engine, then you can write your application using a combination of meta tags and Javascript. As you can see if you're familiar with how PocketBrowser works you are already familiar with how the shared runtime works.
I have previously written a blog on obtaining the shared runtime from the RhoMobile Suite download, you can see it at https://developer.motorolasolutions.com/community/rhomobile-suite/docs/developer-reference/blog/2012/06/29/accessing-the-shared-runtime
If you are interested in porting your Web Application directly to a native Application using RhoMobile Suite you can see a good video on our presentation at our Americas Application Forum here https://developer.motorolasolutions.com/docs/DOC-1636
Porting an application in RhoElements 1.x, RhoElements 2.0 or RhoElements 2.1
RhoElements was designed from day one to be completely backwardly compatible with applications written for PocketBrowser, if you are running RhoElements 1.x, RhoElements 2.0 or RhoElements 2.1 you should be able to point your RhoMobile Suite shared runtime at your existing PocketBrowser application and it should run without modification, though because a different rendering engine is being used underneath you may need to modify your user interface slightly to accommodate.
Backwards compatibility is accomplished in 2 ways:
- PocketBrowser syntax Meta tags and Javascript calls are converted to RhoElements syntax by the use of regular expressions, eg
is converted to
using the Regular expression:
All of the regular expression patterns used by RhoElements can be found in your Shared runtime installation at \Program Files\RhoElements\Config\regex.xml (by default) with separate regular expressions being provided for the HTTP-Equiv components and Content components of the meta tags. This is all done internally meaning RhoElements will see syntax it recognises even though it has been declared in PocketBrowser.
This syntax conversion also applies to calls made through JavaScript.
- ActiveX has been replaced by NPAPI. Remember the PocketBrowser syntax for enabling the Scanner:
var myObj = new ActiveXObject("PocketBrowser.Generic");
myObj.invokeMETAFunction('Scanner','enable');
The WebKit engine (HTML5 rendering engine) used by RhoElements does not understand what an 'ActiveX' object is by default, this this is a Microsoft technology; for this reason the functionality is simulated within RhoElements using an NPAPI object. For more information on NPAPI as well as writing your own I have written a blog post at https://developer.motorolasolutions.com/community/rhomobile-suite/docs/developer-reference/blog/2012/04/10/creating-an-npapi-for-rhoelements. One word of advice though: custom NPAPI creation is only supported on Windows Mobile devices and will continue to be moving forward but with the introduction of consumer device support in RhoElements we are not able to offer NPAPI support across our full range of devices.
There are several configuration options which are appropriate to mention here:
The NPAPI Preloads section of the config.xml file specifies which of the PocketBrowser ActiveX objects should be preloaded on the page, by default they are all preloaded. The NPAPI Directory option specifies which directory the NPAPI objects can be found in, by default on Windows this will point to a sub directory off of where you installed the shared runtime.
Porting an application in RhoElements 2.2
Applying a series of regular expressions to every EMML tag parsed introduces a performance hit whenever a device capability is accessed, if you are using tags or JavaScript functions in your body onLoad() handler the navigation performance of RhoElements will suffer. In RhoElements version 2.2 the decision was made to disable regular expressions by default, this means that if you install the RhoElements 2.2 shared runtime and point it at your existing PocketBrowser application it will not work out of the box. There is a configuration option you can modify to turn regular expressions back on again, unsurprisingly named:
However doing this is inefficient as you will be applying ALL conversions. Towards the end of my second performance blog (https://developer.motorolasolutions.com/community/rhomobile-suite/docs/developer-reference/blog/2012/10/08/rhoelements-performance-improvements-2) I spoke about how the regular expressions applied to EMML tags were stored in an XML file as part of the shared runtime installation, what we want to do is to reduce the default RegEx.xml file containing about 330 conversions in total to only contain the conversions used by your application.
For example, to take the earlier example of an application which used
with no other functionality, though somewhat of a contrived example we could reduce the RegEx.xml file to:
which means we would only be processing one regular expression per EMML tag which is far more efficient.
Of course it's not immediately obvious which PocketBrowser specific EMML calls you are using, your application could have hundreds of calls out to the device capabilities and it may not be obvious which entries in RegEx.xml are being applied. To make reducing your RegEx.xml file easier in RhoElements 2.2 a log entry will be written with severity:Info whenever a regular expression conversion is applied, for example if the example above is found in an application the log will output:
A Regular Expression has been applied to the Module method or parameter (x=50 => left:50)
RegEx.xml entry is "
"
By running your PocketBrowser application in RhoElements 2.2 shared runtime with
Rewriting your application for RhoElements 2.2
So let us suppose you are updating your PocketBrowser application as you move to RhoElements, there are a number of reasons you might want to do this:
- You want to improve your user interface to take advantage of the HTML5 compliant rendering engine.
- You want to access device APIs not available in PocketBrowser
- You wish to transition to a new framework which, detecting the existence of ActiveXObjects on the page, mistakenly serves you up pages configured for Internet Explorer.
It is recommended you follow the following steps:
- Locate every instance of 'ActiveX' in your code and replace it with the corresponding Javascript interface, For example the earlier code snippet
var myObj = new ActiveXObject("PocketBrowser.Generic");
myObj.invokeMETAFunction('Scanner','enable');
becomes
scanner.enable();
and
var myPrinter = new ActiveXObject("PocketBrowser.NarrowBand");
myPrinter.PSExternalEx(257, 'Hello World');
becomes
apd.PSExternalEx(257, 'Hello World');
2. Modify your NPAPI preloads section as follows to stop loading any pseudo-ActiveX objects on the page:
3. Convert all your EMML1.0 syntax to EMML1.1. You can use the technique described in the previous section to determine which of your calls are using EMML 1.0 syntax and the log file will also tell you the corresponding EMML 1.1 syntax.
For example
wrote the following to the log file:
A Regular Expression has been applied to the Module method or parameter (x=50 => left:50)
RegEx.xml entry is "
"
and so it should be converted to
Your upgrade from PocketBrowser to RhoElements is now complete.
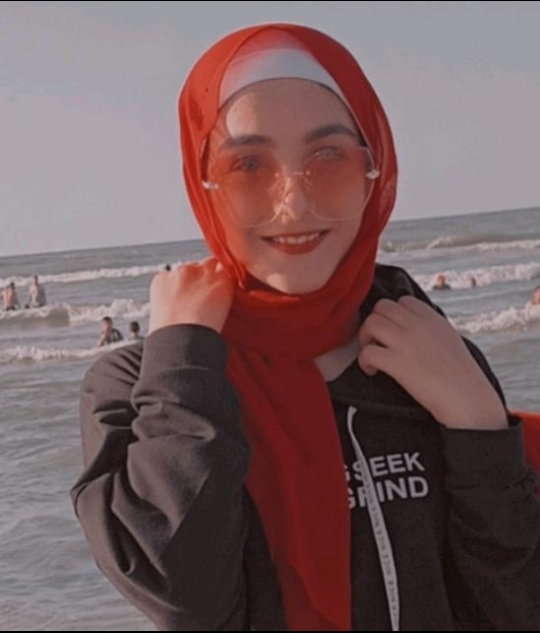
Anonymous (not verified)
Dfghjkjjn
1 Replies
Our company developed a hosted, pure HTML/EMML application for PocketBrowser specifically for the MK3000 this past winter.
Three weeks after product release we were notified that the MK3000 had been discontinued in favor of the MK3100.
RhoElements doesn't have support for the MK3100 until 4.0, which I found the beta for. However, the MK3000 included PocketBrowser without the purchase of an additional license.
Motorola support told us that Rho 4.0 wouldn't even be available until 4th/1st quarter. We've got customers lined up to buy our solution and we can't give it to them.
Is it possible to run the shared runtime without an additional license just to access our current HTML/EMML application? If so, how do we get a license for the beta version?
I just want what PocketBrowser always did, and I don't want to have to charge our customers more for it. I need a solution now.