The fast way to multiplatform data-aware mobile applications. Part 1.
In this multi-part series, you will see how quick and painless it is to build a sophisticated mobile application with two-way data synchronization, using Rhomobile Suite. No prior knowledge is required other than basic HTML and Ruby, the only prerequisite is to have Rhomobile Suite properly installed on your system.
Completing this tutorial will take you approximately 30 minutes, we will start from scratch and by the end of this first post you will already have developed a multiplatform mobile application that gets its data automatically from a server.
Today we are going to start building a twitter search client that will keep everybody in your company up to date on important topics. This, of course, is only an excuse to show you concepts and techniques that are intentionally applicable to other data sources so that you can start building data-heavy enterprise apps today.
Step 1. Create a Rhomobile application.
To get started creating a Rhomobile application you can use the integrated wizards in Rhostudio:
If you prefer the command line instead, it is equally easy:
$ rhodes app tweetstream
Both approaches will result in a new, almost blank application being created, let’s see what it looks like. We will run the application under the Rhosimulator debug environment. It will not make a difference right now whether we “run” or “debug” it, the steps are basically the same, but in the future we will want to set breakpoints and inspect variables, and knowing how to do it from the start will save us time later.
Within Rhostudio, right click the project, click “Debug As...” and click “Debug configurations”...
Create a new “Rhomobile Application” debug configuration
Apply your changes, click Debug and you will get something like this:
If you are using a different IDE or a text editor, you can also start the simulator from the command line with:
$ rake run:iphone:rhosimulator_debug
Step 2. The fun begins
With almost no effort, we already have a native-looking base application and we can start to add our own code to it. What our application is going to do is get a list of twitter searches from a server, together with their related tweets, and we will want to see everything directly in our home screen as soon as the application starts.
In order to customize our home page, we are going to create a new folder under /app called Home and we will add two files there:
- copy /app/index.erb into /app/Home/index.erb
- create a new file called /app/Home/home_controller.rb with the following content:
require 'rho/rhocontroller'
require 'helpers/browser_helper'
class HomeController Rho::RhoController
include BrowserHelper
# GET /Home
def index
render
end
end
This controller is as simple as it gets, it will not do much yet, but it is enough to have the application render the Home screen.
In order to have our new controller be the starting point of the application when it opens, we need to make a small change to the rhoconfig.txt file. At the very beginning of that file you will see an entry for start_path, update it so it looks like this:
# startup page for your application
start_path = '/app/Home'
Here, "Home" matches the directory we created earlier and where we put our files. The name is not important, you could have called it anything else, but Home is descriptive for this purpose.
If we run the application now, not much will have changed from the first time. We will see the same screen as before, but now we are ready to start adding some of our own code.
Update home_controller.rb so that the index method looks like this:
def index
# we do not yet have a connection to the server, so we will test with hardcoded data
@searches = [
{:id => "1", :query => "rhomobile"},
{:id => "2", :query => "#fun"}
]
render
end
Great, now we have some data we can test with, but they still do not appear anywhere we can see. As in any application, we need to update the view to show what we need: open /app/Home/index.erb, find the div with data-role=”content” and edit it to match the following:
% @searches.each do |search| %>
- %= search[:query] %>
% end %>
Try to run the application once more and we should start to see see some changes
That looks better, but we are only displaying local data and our goal is to receive our information from a server; let’s see what must be done to make our application interact with the outside world. The part of RhoMobile Suite in charge of synchronization is Rhoconnect. This framework does all the heavy lifting for us, taking care of two-way data synchronization and it only asks of us that we do two things:
- Add data models to our mobile app. This lets the app know which data it has to sync
- Write a small adapter so that RhoConnect can interface with our data source. This code takes data from an external data source, be it a database, ERP system or any other service you can think of and formats the data for consumption by the mobile app
Let’s get started: create a new Rhoconnect application and call it “tweetserver”
Now, create a “Source adapter” called “Search”:
Or from the command line:
$ rhoconnect source Search
It is in this source adapter that our part of the magic will take place. Open /sources/search.rb, find the “query” method and change it like this:
def query(params=nil)
@result = {
"SEARCH_1"=>{:query => "RhoMobile"},
"SEARCH_2"=>{:query => "#fun"}
}
end
Add another source adapter called “Tweet”, and update its query method:
def query(params=nil)
@result = {
"1" => {:search_id => "SEARCH_1", :status => "Rhomobile lets you leverage HTML and ruby skills for mobile development"},
"2" => {:search_id => "SEARCH_1", :status => "This is a hardcoded tweet but at least it came from a server"},
"3" => {:search_id => "SEARCH_2", :status => "Lorem ipsum dolor"},
"4" => {:search_id => "SEARCH_2", :status => "consectetuer adipiscing elit"}
}
The "query" method is called automatically by the RhoConnect synchronization engine whenever a client (our mobile app) asks if there is new data to retrieve and what RhoConnect expects us to return is a hash of hashes. In an enterprise application, instead of returning hardcoded values, we would connect to our database and issue SQL statements, or maybe call a REST API, and return the results. We will see an example of interfacing with external data servers in a future post of this series.
Start this RhoConnect instance, make sure it’s running and go back to the mobile app.
$ rhoconnect start
Now we have a server ready to distribute data, but our app does not yet know that. The second step to achieve data synchronization is to add a data model to the mobile application:
If you prefer the command line, instead of using the wizards run:
$ rhodes model Search query
Where “Search” is the name of the model and “query” is the only attribute this model is going to have for now.
Once the model is generated, we need to tell it that we want to use data synchronization: open Search/search.rb and uncomment the line that says “enable : sync”. That’s it, our mobile application will now sync searches with the server, without us having to write any code.
Now that the application knows how to get search data from a server, let’s update our home screen to remove our hardcoded values and instead display what the server gives us.
Open /app/Home/home_controller.rb, remove the hardcoded values from the index method and update it to contain the following:
def index
@searches = Search.find_all
render
end
What did we just do?
- Rhomobile Suite includes Rhom, an object-relational mapper that helps us with Create, Retrieve, Update and Delete operations.
- Search is the model we generated in the previous step, and thanks to Rhom, it comes with a few built-in methods to operate on data and make our lives easier.
We want to show every search on our home screen, so the find_all method is all we need, and we do not even need to write SQL. Before it can connect with the server, the application must know where that server is located. Open rhoconfig.txt and, around line 50, you will find
syncserver = ‘’
simply change that to read:
syncserver = ‘http://localhost:9292/application’
There is one more small change we must make in /app/Home/index.erb. Instead of
%= search[:query] %>
now that we have a proper model we want to have
%= search.query %>
We are almost there, but if we run our application now, the home screen will still not show any searches. Why? Because we still have not established a connection to the server. Click the “Login” button, enter “user1” as the login (anything will do, actually), leave the password empty and click “Login”.
Voila! we almost magically have data there now and we didn’t write any synchronization code. Isn’t enterprise mobile app development fun?
Now that we have the searches, let’s also show some tweets. Add another RhoMobile model called “Tweet” with a single attribute called “status” and, in the generated “Tweet/tweet.rb” file, uncomment “enable : sync” like we did previously for Search
The application will synchronize Searches AND Tweets now, let’s update the home screen to show them.
Open /app/Home/index.erb again and update the code:
% @searches.each do |search| %>
- %= search.query %>
% tweets_for_search(search).each do |tweet| %>
- %= tweet.status %>
% end %>
% end %>
We just added a new list under each search, to display its associated tweets by means of a new method we called “tweets_for_search”. Let’s go back to /Home/home_controller.erb in order to add the tweets_for_search method:
def tweets_for_search(search)
tweets = Tweet.find_all(
:conditions => {
{
:name => :search_id
} => search.object
}
)
tweets
end
What this method says is, “Find all tweets where the search_id is the current search”.
Run the application again and, lo and behold, there we have our searches and their tweets, all nicely displayed. While we invoked a few wizards and configured a few settings, the underlying RhoMobile platform did all the heavy lifting of data synchronization for us. That is a lot of code we do not have to write, debug or maintain and is a core part of the platform's value proposition
What’s next?
We are receiving data from a server, but we have not seen yet how to upload our own data to it. In the next installment of this series we will see how to create data in our mobile application and distribute it to the server and other clients.
About Us:
Kutir Mobility is your partner in the enterprise mobile app space, empowering your team with our custom training sessions and complementing it with our own in-house development expertise. Get in touch today to schedule a free consultation with one of our mobile architects.
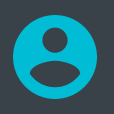
Kutir Mobility
1 Replies
This is not clear how to execute "QUEUE=tweetfetcher rake resque:work". Please clarify.