Getting Printer Alerts Using HTTP-POST Messages
In this blog, we'll explain how to configure the printer and how to build a listener and an error viewer pages in PHP with a MySQL database on the back to store the alerts.
All the Link-OS printers support the HTTP-POST protocol to send messages from the printer to a webserver. These messages contain information about the printer status and possible error status, so, if correctly used, this feature can be used to monitor a fleet of printers.
Pre-requisites
- A LinkOS printer
- A web server with PHP and MySQL
- Basic PHP and MySQL knowledge
Step 1
Let’s first configure the printer to send the messages to the web server.
The type of messages supported are the following:
- ALL MESSAGES
- PAPER OUT
- RIBBON OUT
- HEAD OPEN
- RIBBON IN
- CUTTER JAMMED
- PRINTER PAUSED
- MEDIA LOW
- RIBBON LOW
- BATTERY LOW
- INVALID HEAD
- COUNTRY CODE ERROR
- BATTERY MISSING
- MEDIA CARTRIDGE
- MEDIA CARTRIDGE LOAD FAILURE
- MEDIA CARTRIDGE EJECT FAILURE
- MEDIA CARTRIDGE FORCED EJECT
For a matter of simplicity, this blog will use the option to receive all the messages, but you can narrow down the list and select only the messages you really need.
In order to configure a printer, you need to send to it the following commands
! U1 setvar "alerts.configured" "COLD START,SNMP,Y,N,255.255.255.255,162,N"
! U1 setvar "weblink.restore_defaults" ""
! U1 setvar "alerts.add" "ALL MESSAGES,HTTP-POST,Y,Y,http://www.example.com/postlistener.php,0,N,"
! U1 setvar "device.reset" ""
The first 2 commands are only required to be sure the printer is set to the default configuration in regards of the settings we're going to use for this solution, while the 3rd is the actual configuration command to enable the alerts and the last is just to reboot the printer so it can apply the changes.
If you want to only receive alerts for “paper out” and “ribbon out” errors, for example, you’ll have to send the following command, one for each type of alert
! U1 setvar "alerts.add" "PAPER OUT,HTTP-POST,Y,Y,http://www.example.com/postlistener.php,0,N,"
! U1 setvar "alerts.add" "RIBBON OUT,HTTP-POST,Y,Y,http://www.example.com/postlistener.php,0,N,"
Once the printer will be rebooted, it will start to send the alerts.
Step 2
Assuming you have a webserver which supports PHP and MySQL, you should create (or already have) a file which manages the connection to the database, something like the following one
conn.php
$servername = "localhost";
$username = "root";
$password = "password";
$dbname = "http-post";
$conn = new mysqli($servername, $username, $password, $dbname);
mysqli_query($conn,"set names 'utf8'");
So you then need to create a database called "http-post" and import the following dump of an empty table which already includes all the required fields to store the alerts
-- phpMyAdmin SQL Dump
-- version 4.9.7
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
SET AUTOCOMMIT = 0;
START TRANSACTION;
SET time_zone = "+00:00";
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8mb4 */;
--
-- Database: `http-post`
--
-- --------------------------------------------------------
--
-- Structure of the table `logs`
--
CREATE TABLE `logs` (
`id` int(100) NOT NULL,
`sn` varchar(20) NOT NULL,
`alerttype` varchar(50) NOT NULL,
`alertmessage` varchar(50) NOT NULL,
`date` timestamp NOT NULL DEFAULT current_timestamp() ON UPDATE current_timestamp()
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- Indexes for the table `logs`
--
ALTER TABLE `logs`
ADD PRIMARY KEY (`id`);
--
-- AUTO_INCREMENT for the table `logs`
--
ALTER TABLE `logs`
MODIFY `id` int(100) NOT NULL AUTO_INCREMENT;
COMMIT;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
Step 3
Once the printer is configured, the database is ready and we have configured the connection to it, we need to build the listener page.
Check the comments in the page for more details.
postlistener.php
// get the alert message from the POST message
$alertMsg = urldecode($_POST["alertMsg"]);
// get the printer serial number
$sn = urldecode($_POST["uniqueId"]);
// since all the messages are in the format "type: message"
// this command splits the type of message and the message content into 2 different variables
list($alerttype, $alertmessage) = explode (": ", $alertMsg);
// including the PHP file which manages the database connection
include "conn.php";
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// editing the messages into lower case
$alerttype = ucfirst(strtolower($alerttype));
$alertmessage = ucfirst(strtolower($alertmessage));
// query to insert the message into the database
$stmt = $conn->prepare("INSERT INTO logs (sn, alerttype, alertmessage) VALUES (?, ?, ?)");
$stmt->bind_param("sss", $sn, $alerttype, $alertmessage);
$stmt->execute();
$stmt->close();
$conn->close();
And the following file just pulls the data from the database and creates a table to show them
postview.php
// creating a table to show the results
echo “<table border='1' width='75%'>
<th>Date</th>
<th>Serial number</th>
<th>Alert type</th>
<th>Alert message</th>”;
// including the PHP file which manages the database connection
include "conn.php";
// querying the database to show everything in the table called "logs"
$query = "SELECT * FROM logs";
// fetching the result from the database into a multidimensional array
$result = mysqli_query($conn,$query);
// fetching the array into single rows and reiterating this operation to show the data
while($row = $result->fetch_assoc()) {
echo "<tr>";
echo "<td>" . $row["date"] . "</td><td>" . $row["sn"] . "</td><td>" . $row["alerttype"] . "</td><td>" . $row["alertmessage"] . "</td>";
echo "</tr>";
}
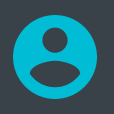
Mattia Pappagallo
0 Replies