Using the HD4000 SDK With Xamarin
Introduction
As some of you may have seen earlier this year, we released a three-part series on developing applications for the HD4000 on Android (you can find that here). Well, following that, we can now officially confirm our HD4000 SDK supports the Xamarin development environment too! This blog will walk you through the steps of creating a new project, importing the SDK and the configuring the SDK to work with your application.
Creating your Project
As always, the first thing we’re going to need to do is create a new project. I’ll assume you’re familiar with this step however for clarity I’ve used the following configuration
Name: HD4000XamarinSDKDemo
Template: Blank App (Android)
Min SDK: Lollipop 5.0 (API 21) – This is the minimum Android API supported by our SDK
Once complete, your IDE should look something like this:
Importing the SDK
The next step is to import the SDK .aar file into our project. We do this by using the Android Bindings Library template, making sure to set the build action to “LibraryProjectZip”. Microsoft have documented this process here if you need some further info, otherwise, please read on to see this broken down step-by-step.
- Create a new Bindings Library project (right-click Solution > add > new Project), using the Android Bindings Library Template – note: I’ve named my binding HD4000Binding, but you can change this if you like:
- We should now see a new project in our Solution, in my case HD4000SDKBinding. Inside this project is a Jars folder, where we need to add our SDK .aar File. Simply right-click on the Jars Folder > Add > Existing Item, then navigate to the SDK .aar File on your development machine – your project should now look something like this:
- Next, we need to set the Build Action to “LibraryProjectZip” – we can do that by right-clicking the .jar > properties > set Build Action to “LibraryProjectZip”:
- Finally, we need to create a reference to this binding from our project. Simply right-click on references folder in your project, then select “add reference”. Under projects you should see your SDKBinding Project, select the checkbox and tap ok:
- You can test this has worked by double clicking the project under the references folder, this should open the Object Browser and you should be able to see the classes contained within our HD4000 SDK.
Adding Dependencies
In order to use our SDK, there’s a few dependencies you need to include in your project. Navigate to the NuGet Package Manager Console (tools > NuGet Package Manager > Nuget Package Manager Console) and import the following packages into your project:
Install-Package Xamarin.Kotlin.StdLib -Version 1.3.72
Install-Package GoogleGson -Version 2.8.5
Install-Package Xamarin.AndroidX.Core -Version 1.3.0.3
Install-Package Xamarin.AndroidX.Lifecycle.LiveData -Version 2.3.0.2-alpha03
Install-Package Xamarin.AndroidX.Legacy.Support.Core.UI -Version 1.0.0.5
Install-Package Xamarin.Google.Android.Material -Version 1.1.0.5-rc3
Install-Package Xamarin.AndroidX.Browser -Version 1.3.0.3-alpha01
Implementing the SDK
So far, we’ve created a new project, imported the SDK into that project, and added the required dependencies to support the SDK. The last thing we need to do before we can start using the SDK is implement the relevant life-cycle call backs
Implementing these lifecycle callbacks lets the SDK know what state your application is in, so that the driver can handle the connection to the HD4000 appropriately, i.e. displaying an interrupt message when your application is backgrounded, for example. The four lifecycle methods we need to implement are: onStart, onStop, onPause and onResume, calling the respective methods from the SDK inside these callbacks, like so:
public class MainActivity : AppCompatActivity, ZebraHud.IEventListener
{
// Create HUD
ZebraHud hud;
protected override void OnCreate(Bundle savedInstanceState)
{
…
// Create HUD
hud = new ZebraHud();
}
protected override void OnStart()
{
base.OnStart();
this.hud.OnStart(this);
}
protected override void OnResume()
{
base.OnResume();
this.hud.OnResume(this, (ZebraHud.IEventListener)this);
}
protected override void OnPause()
{
base.OnPause();
this.hud.OnPause(this);
}
protected override void OnStop()
{
base.OnStop();
Java.Lang.Boolean showInterrupt = (Java.Lang.Boolean)true;
this.hud.OnStop(this, showInterrupt);
}
/**
* ZebraHud.IEventListener Callbacks
*/
void ZebraHud.IEventListener.OnCameraImage(Bitmap p0)
{
Log.Info("HUD", "New Image");
}
void ZebraHud.IEventListener.OnConnected(Java.Lang.Boolean p0)
{
Log.Info("HUD", "Connected: " + p0);
}
void ZebraHud.IEventListener.OnImageUpdated(byte[] p0)
{
Log.Info("HUD", "New Image");
}
}
}
The eagle eyed will have noticed that we also implemented an interface in our class: ZebraHud.IEventsListener – it is not required to implement this interface, but doing so provides you access to connection notifications, as well as access to the Camera.
At this point, we’re ready to start developing our application! If you’re interested in learning a bit more about developing apps using our SDK, you can check out part 1 of our 3 part guide here: https://developer.zebra.com/blog/developing-android-apps-hd4000-part-1-getting-started
Finally, I’d like to give a big thanks to Nicola De Zolt Lisabetta for his help in putting together this blog!
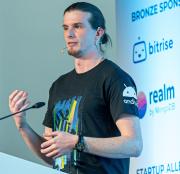
James Swinton-Bland
Replies