Enterprise Android in a Hosted Cloud IoT Solution: Part 3: Amazon Web Services (AWS)
This is part three in a series of blog posts looking at integrating Zebra Android devices as IoT clients in a public cloud infrastructure. For other posts in this series, please see the links below:
- Part 0 – Enterprise Android in a Hosted Cloud IoT Solution: Introduction
- Part 1 – Google Cloud IoT Core Data Processing
- Part 2 – Interfacing a Zebra mobile computer with Google Cloud IoT
- Part 3 – Amazon Web Services (AWS) IoT Core Data Processing
- Part 4 – Interfacing a Zebra mobile computer with AWS IoT
- Part 5 – Microsoft Azure IoT Hub Data Processing
- Part 6 – Interfacing a Zebra mobile computer with Azure IoT Hub
This post will walk through an example cloud IoT infrastructure using Amazon Web Services: IoT as the entry point. An MQTT client will mimic device location, Android OS info and battery information and this data will be stored in a database for later retrieval. The next part in this series will move to using a real device.
This example is based loosely on Ed Lima’s excellent post on implementing an IoT backend with AWS Lambda & DynamoDB
Disclaimers:
- This is a very simple example, but the aim is to show the principles of subscribing to an MQTT topic, taking action on data posted to that topic by an IoT client and storing the IoT data for later retrieval and analysis.
- Any hosted cloud computing solution may incur costs, please ensure you are aware of the costs associated with the cloud components you are using and how to monitor and set spending limits.
Objectives:
- Publish simulated device telemetry data to an MQTT topic
- Use AWS IoT rules to trigger specific logic in response to posts on an MQTT topic
- Deploy an AWS lambda function to subscribe to the telemetry data and store the received values in an AWS DynamoDB
- Visualize low battery events from any device providing data
- Data that will be simulated along with some sample values, defined in JSON:
{
"deviceId": "Test-device",
"dateTime": "2018-11-14T10:29:45",
"model": "TC57",
"lat": "35.6602997",
"lng": "139.7282743",
"battLevel": "20",
"battHealth": "50",
"osVersion": "8.1.0",
"patchLevel": "2019-02-01",
"releaseVersion": "01-10-09.00-OG-U00-STD"
}
Source Code:
The source code discussed in this tutorial is available from GitHub.
Pre-requisites:
- Familiarity of AWS and AWS IoT, Lambda functions and DynamoDB services are useful but not required for this tutorial. For more information on these topics, see the following links:
Configure an AWS project
- Navigate to https://console.aws.amazon.com and sign in with an Amazon account
Create a role with DynamoDB the required ‘execution role’ on DynamoDB
This will allow the lambda function to store data in the DynamoDB that it receives from the MQTT topic.
- Open the IAM Management console
- Select roles
- Choose ‘Create role’
- Choose the ‘Lambda’ service (allows Lambda functions to call AWS services on your behalf)
- Choose ‘Next: Permissions’
- Choose ‘AmazonDynamoDBFullAccess’ and ‘AWSLambdaBasicExecutionRole’
- Choose ‘Next: Tags’. Do not assign any tags
- Choose ‘Next: Review’.
- Assign a role name (e.g. store-data-role)
- Choose ‘Create Role’
Create the Lambda function to insert new device data into the database
This function will be called when data is received on the MQTT topic. The function will store received data in a DynamoDB table and console log when a low battery condition is seen.
- Open the Lambda console
- Choose ‘Create function’
- Assign a function name (e.g. store-device-data)
- Choose a Node.js runtime
- Chose the ‘Existing role’ you created in the previous step, in this case ‘store-data-role’
- Choose ‘Create function’
- Add an AWS IoT trigger to the function
- Scroll down to Function code
- Enter the following function code, available from GitHub.
console.log('Loading function');
var AWS = require('aws-sdk');
var dynamo = new AWS.DynamoDB.DocumentClient();
var table = "device-data";
exports.handler = function(event, context) {
//console.log('Received event:', JSON.stringify(event, null, 2));
var params = {
TableName:table,
Item:{
"deviceId": event.deviceId,
"dateTime": event.dateTime,
"model": event.model,
"lat": event.lat,
"lng": event.lng,
"battLevel": event.battLevel,
"battHealth": event.battHealth,
"osVersion": event.osVersion,
"patchLevel": event.patchLevel,
"releaseVersion": event.releaseVersion
}
};
console.log("Received device data");
dynamo.put(params, function(err, data) {
if (err) {
console.error("Unable to add device. Error JSON:", JSON.stringify(err, null, 2));
context.fail();
} else {
console.log("Received device data:", JSON.stringify(data, null, 2));
context.succeed();
}
});
}
- Scroll down and choose the IoT type ‘Custom IoT rule’
- Choose to ‘Create a new rule’
- Assign the rule a name, e.g. storeDeviceDataRule
- Define the query statement as “ SELECT * from ‘deviceTelemetry’ “
- Choose Add and Save
- The completed rule should look something like this
Create the DynamoDB table
The DynamoDB will hold received device data
- Open the DynamoDB console
- Choose create table
- Define a table name, e.g. device-data
- Define the primary key as ‘deviceId’ and the sort key as ‘dateTime’
- Click ‘Create’
- The table will be created. You can view the items in the table by selecting the ‘Items’ tab.
Test data is being stored
Testing MQTT is very easy with AWS thanks to the inbuilt test client.
- Return to the AWS IoT home
- Select ‘Test’ to launch the MQTT client
- Publish to the topic ‘deviceTelemetry’ (you can copy / paste the sample code from the top of this post)
- Returning to your DynamoDB table, you should see the data has appeared
- Be sure to modify the dateTime or deviceId attributes on subsequent messages to ensure the primary key is unique.
Visualizing low battery events
Because we performed a console log in the Lambda function whenever a low batter condition was seen (15% or less), we can create a rudimentary view of this as a log insight:
- Open the AWS CloudWatch
- Select Logs Insights
- Select the previously created lambda from the dropdown, in my case ‘store-device-data’
- Add the following query:
fields @message
| filter @message like /BATTERY LOW/
- Run the query to see any devices which have reported a low battery level
There would be many superior ways to query low batteries, for example extracting the levels from the DynamoDB table but the above technique may well be sufficient for small / medium volume installations.
Debugging
If something does not work after you have performed the above steps, try using AWS CloudWatch to determine what might be causing the error.
You will need to make sure you have the “AWSLambdaBasicExecutionRole” policy associated with the store-data-role you created earlier in the IAM Management console
Conclusion
The astute observer will note that we have not actually created an IoT device in AWS during this tutorial but have used an IoT rule and MQTT topic to perform the core functionality of data collection. The next step in this post series will integrate with a physical Android device and set up the IoT shadow at that stage which would allow for communication with and control of the IoT device.
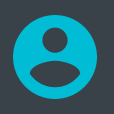
Darryn Campbell