Location APIs available on GMS & AOSP devices
Zebra offers a multitude of locationing solutions for a huge range of customers and use cases. Technologies such as Real time locatating system (RTLS) which includes Dart UWB and WhereNet, both of which use dedicated hardware tags and sensors alongside technology based on existing standards to provide high accuracy for high value assets. Although it is available worldwide, in North America you will have seen this technology prominently featured as it is used in the NFL . Zebra also offers the recently launched Smartlens targeting retail use cases and using a variety of technologies including RFID and Wi-Fi feeding data into an analytics engine to provide complete retail insight. RFID itself can of course be used to determine an asset’s location and Zebra have a wide variety of printers and readers for this purpose.
All these technologies are great and can of course be supplemented by technologies ubiquitous in consumer deployments such as Bluetooth Low Energy (BLE) beacons and GPS, indeed providing visibility into your entire organization workflow is a key pillar in Zebra’s corporate objectives so expect to see additional innovation and harmonization in this area in the future.
With such a huge scope it is not possible to cover everything in detail in a single blog. This blog is aimed solely at developers wishing to use the location technologies available on Zebra android devices and provides some of the special considerations to take when targeting enterprise hardware. Technologies like GPS, Wi-Fi, BLE and cellular based location are the fundamental building blocks on which many location-aware applications are built so it is important to understand what is available and any dependencies.
Android APIs
Critical to understanding Android’s location APIs is appreciating that there are two entirely separate but related APIs available for use in your application:
The Platform location API (android.location and Locationmanager) is available on all Android devices offered by Zebra, both AOSP (Android Open Source Project) and GMS (Google Mobility Services). These are coloured blue in the diagram below.
The Google Play services location APIs (com.google.android.gms.location) are Google’s recommended way to determine device location but since they are part of Google Play services, they will only run on GMS devices. These are coloured red in the diagram below.
Another set of APIs which depend on Google Play services are known as the Awareness APIs, these are partly cloud-based APIs which also only run on GMS devices and are also coloured red in the diagram below.
This blog goes into more detail on how to access each of these APIs and their dependencies but the diagram below shows the rough architecture and the options available to a user application.
Location Manager & Providers
The Location Manager class can be used to determine the device location on both AOSP and GMS devices. The manager depends on underlying ‘Providers’ and the code has named constants for GPS, Network and Passive providers:
- The GPS provider gives the location based on the device’s GPS hardware.
- The Network provider gives the location determined from both the device’s cellular connection through tower triangulation and nearby Wi-Fi access points as provided by Google’s own data acquisition & heuristics which can be used to associate Mac addresses to physical location.
- The Passive provider will determine the location as best it can based on location generated by other providers, it is designed for power considerate applications e.g. those running in the background.
Location updates can be requested from any of the pre-defined providers at specified intervals or as a single request but there is no ‘onChanged()’ callback provided for location. The application is responsible for determining which provider is the best one to use and Google have a page in their documentation detailing some strategies but at its simplest an application would likely register with all providers and use the location with the best reported accuracy.
Location Manager also offers a basic type of GeoLocation. You can specify a circular geofence (called a proximity alert (https://developer.android.com/reference/android/location/LocationManager.html#addProximityAlert(double, double, float, long, android.app.PendingIntent)) ) around a location and will be notified when a user enters or exits that location
There are obviously some dependencies when using Location Manager:
- The GPS provider is only available on devices with a GPS chip and to applications which have the ACCESS_FINE_LOCATION permission granted.
- The Network provider requires either the ACCESS_FINE_LOCATION or ACCESS_COURSE_LOCATION permission and can only provide cell-tower based locationing on devices with a cell connection. For the Network provider to be able to issue Wi-Fi based locationing the device settings need to allow for Wi-Fi scanning or have Wi-Fi enabled and the device needs to have an external internet connection to be able to communicate with Google’s backend.
- The Passive provider depends on another application on the device having previously requested location updates and requires the application to have the ACCESS_FINE_LOCATION permission.
Remember from Android Marshmallow onwards the user is empowered at any time to remove your application permissions including ACCESS_COURSE_LOCATION and ACCESS_FINE_LOCATION so ensure you take steps to avoid that. In addition, the device itself also needs to have the location setting enabled with the appropriate mode, as explained later in this blog.
Custom Provider
Android also offers the ability to define a custom provider, this works similarly to the GPS or Network providers but rather than the data being fed by some underlying hardware it is instead fed from whatever source your application chooses to define. In order for your custom provider to initiate correctly you need to select the ‘mock location app’ from the Android developer settings (Android M and higher) or ensure ‘allow mock location’ is checked (Lollipop), both of which can be found under the developer settings.
You can define the name of the custom provider to be whichever Android String you like with the exception of “gps”, “network” and “passive” which are the predefined constants for the built-in providers. Setting your custom provider to any of these names will replace the built-in provider and is the technology underlying various apps in the play store which offer to mock your location either to cheat at gaming or cheat on your significant other (judging by the reviews). Enterprise use cases are somewhat less illicit, perhaps being used to demonstrate a GPS navigation program or test a delivery routing application with a series of pre-defined inputs, indeed Android Studio will give a linting error if you try to use the ACCESS_MOCK_LOCATION permission in a release build manifest.
For purely testing purposes, it is likely the majority of use cases can be addressed by the use of an emulator to playback a series of GPS coordinates to a running application and Google has a short guide to doing this. Since it is not possible to emulate Zebra devices it may be necessary to use a custom provider in order to test an application on real hardware that depends on GPS navigation. In my experience, I have found it is always a good idea to reset the device after you have finished using any custom provider.
The Fused Provider & Location Services
The Google Play services location APIs (com.google.android.gms.location) will nearly always provide a superior experience to the Location Manager APIs discussed in the previous section, giving a location faster and to a greater level of accuracy when tested on the same device. Because the services location APIs depend on Google Play services, they are only available on GMS devices and require location services to be enabled which is typically done as part of the start-up wizard when the device is first initialised (see later section).
Keeping with the provider nomenclature, the GMS based location APIs provide a ‘Fused’ location provider but this offers a different interface than the GPS or Network providers requiring you to first connect to and manage the lifecycle of the GoogleApiClient running on the device. You are still limited to requesting updates at a specified frequency but with the fused provider you have more options how you receive the location callbacks e.g. PendingIntent or LocationListener.
Perhaps unsurprisingly, the fused provider automatically fuses all location sources together for you returning its best guess as to the device location. Recall how with the Location Manager the developer had to determine the best location source from GPS or Network depending on accuracy, age and a number of other factors; this burden is taken away from the developer using the fused provider. It is not possible to influence the fused provider with the Location Manager custom provider described previously but the fused provider does offer a setMockLocation method (https://developers.google.com/android/reference/com/google/android/gms/location/FusedLocationProviderApi.html#setMockLocation(com.google.android.gms.common.api.GoogleApiClient, android.location.Location)) which performs a similar purpose.
Geofencing, Activity Recognition & Reverse Geocoding
Geofencing offered by the GMS-based APIs is slightly more powerful than that found in the Location Manager. You can now register to receive callbacks whenever a user is loitering in a region and therefore supports an expanded set of use cases for example a package delivery taking longer than expected.
The Activity Recognition API provides Android’s best guess what the user is doing, either: In a vehicle, on a bicycle, on foot, running, being still, tilting the device or walking (The full list is here). In my experience, this is very accurate and perhaps most powerfully all calculations are done on-device meaning if the device does not have a network or cloud connection you can still take advantage of this API to determine user activity.
The Android Geocoder API is also only available on GMS devices with location services although it does not depend on the GoogleApiClient like Geofencing and Activity Recognition. The Geocoder class offers both geocoding and reverse geocoding meaning it will return a lat / long based on an address and vice-versa. In my experience the API provides very accurate results and it has clear benefits to package delivery use cases.
Google Awareness API
The Google Awareness APIs are part of play services and are designed as a “unified sensing platform” enabling actions to be triggered when various criteria are met or to provide an overall snapshot of the user’s current environment. The overlap with location comes from how the API defines the user environment and which criteria can be used to trigger context aware actions.
Since the Awareness API depends on a Google backend you will need to have an API_KEY included in your application which, depending on the intensity you plan on using the API, might be a paid service to Google. Additional keys are required to make use of the Places and Beacons features.
The following information about the user’s environment is made available through the awareness API:
Environment | Comments |
---|---|
Position | This will return the same values as the fused location provider from location services and it has the same requirements: The device needs to have the appropriate settings enabled and the application needs to have the required permission to retrieve device location. Since the Awareness API is not designed primarily with location retrieval in mind, applications wishing to retrieve periodic location updates should instead use the previously described location services API. |
Activity | This is equivalent to the Activity Recognition function of location services and when run on the same device, will return the exact same values. Also like the equivalent function in location services, this API will work in a disconnected environment, depending on device accelerometers to determine what the device is doing which can be powerful in many enterprise use cases for example detecting theft if a warehouse-based device is ‘in a car’. |
Nearby Places | This is unique to the Awareness API providing a list of physical locations nearby using Google’s vast repository of map data and as such requires an external internet connection. In my experience, the places highlighted range from business to community areas which would prove useful in delivery use cases however since the data is backed by Google, your experience may vary elsewhere in the world, particularly as much of the data will be crowd sourced. |
Beacons | The Awareness Beacon API is designed to be used exclusively with the Google Beacon Platform which is good if you are planning on using Google’s platform but if you plan on using a third-party beacon system then I do not see any integration options. |
Weather | No equivalent elsewhere in the location APIs. |
Headphones State | No equivalent elsewhere in the location APIs. Since this is only for pluggable headphones the Enterprise use case is somewhat limited as the majority of Enterprises who deploy headphones are using Bluetooth. |
Hardware & Software dependencies
Any locationing technology has a number of hardware dependencies: as previously mentioned GPS requires a GPS chip, network based location requires either a Wi-Fi or WAN device, location based on BLE will require Bluetooth Low Energy on the device compatible and Android Lollipop for the best performance. The following table attempts to give an overview of the hardware available on Zebra’s current crop of Android devices (as well as immediately dating this blog!)
Device | GPS | WAN Capable | Bluetooth | GMS Variant Available | Notes |
---|---|---|---|---|---|
MC18 | No | No | Yes | No | Designed purely as a personal shopping device |
MC36 | Yes | Yes | Yes | No | Device is KitKat only which has early support for BLE. |
MC40 & MC40-HC | No | No | Yes | No | Designed to work within the 4 walls. |
MC9200 | No | No | Yes | No | |
TC51 | No | No | Yes | Yes | Designed to work within the 4 walls. |
TC55 | Yes | Yes | Yes | Yes | |
TC56 | Yes | Yes | Yes | Yes | |
TC70 | No | No | Yes | Yes | Designed to work within the 4 walls. |
TC70X | No | No | Yes | Yes | Designed to work within the 4 walls. |
TC75 | Yes | Yes | Yes | Yes | |
TC75X | Yes | Yes | Yes | Yes | |
TC8000 | No | No | Yes | Yes | Designed primarily as a warehouse device. |
Location Settings & Permissions
Device settings
There are a surprising number of device settings associated with location, each of which will need to be configured as part of your staging process to ensure applications requiring location behave as expected.
Setting Name | Description | Screenshot |
---|---|---|
Location On / Off | The most fundamental setting for Android location under Settings --> Location. Must be enabled for any location API to function. | ![]() |
Location Mode | Also found under Settings --> Location the options are ‘High accuracy’, ‘Battery saving’ or ‘Device only’ and the UI is self-explanatory. You will not be given any options if your device lacks a GPS chip. | ![]() |
Wi-Fi scanning (M & higher) / Scanning always available (KK / LP) | This setting allows your device to take advantage of the crowd-sourced AP location available as part of Google’s location service even when your device’s main Wi-Fi is turned off. If your device’s Wi-Fi is turned on the device will scan for nearby APs regardless of this setting. If his setting is turned off and the device Wi-Fi is also turned off then no Wi-Fi based location will be available (the device will still have access to cellular, BLE and GPS location, depending on settings). This option moved between Lollipop and Marshmallow, previously under Wi-Fi --> Advanced it is now under Location --> Scanning (or just search settings for ‘Scanning’) |
Wi-Fi scanning on a KK device |
Bluetooth scanning | This setting is only available on Marshmallow devices or higher and is designed to allow Android to take advantage of BLE beacons to improve the location accuracy of the fused provider (and anything that depends on the fused provider such as Geofencing). This does not impact an application’s ability to scan for BLE devices using the BluetoothLeScanner API or use of the Awareness API. |
Wi-Fi and Bluetooth scanning on an M device |
Google’s Location service On / Off (GMS Only) |
GMS devices require the user to agree to use “Google’s location service” which is the technology underpinning the “fused provider and location services”, covered earlier. There is no check box in the Settings UI, the agreement must happen either as part of the set-up wizard or after setting up the device if participation was declined during setup (image 1). The user will be prompted to agree to the terms of the location service when they turn Location On if they have not already done so (image 2). The Location Services SettingsAPI method can be used as an alternative way to agree to the terms of the location service and image 3 shows the dialog given after a request for high accuracy and BLE on a device without location services accepted. Note the 3rd line item agreeing to the terms of the service. |
Image 1: Sign up as part of the set-up wizard
Image 2: Sign up when enabling location
Image 3: Sign up after being prompted by the SettingsApi |
GPS Xtra | GPX Xtra will only be available on devices whose WAN stack & hardware supports it. It is a Qualcomm specific feature for the GPS implementation on specific devices (including some Zebra devices) and allows GPS information to be accessed from an eXTended Receiver Assistance (Xtra) server using an Intentet connection, enhancing the operation of standalone GPS. | ![]() |
In addition to location based Android settings, there are a number of other device settings which are not specific to location but will nonetheless have an effect on it.
Setting Name | Description | Screenshot |
---|---|---|
Wi-Fi On / Off |
Turns the device Wi-Fi on or off and will take priority over Wi-Fi scanning; when on, Wi-Fi scanning will always be on.
Many location techniques can depend on external data for example the Network provider may need to communicate with Google’s servers to retrieve a location based on nearby Wi-Fi APs. |
![]() |
Bluetooth On / Off | Bluetooth is required by any application wishing to use BLE beacons via the BluetoothLeScanner API or the Awareness API (discussed earlier) | ![]() |
Mobile Data On / Off | Similar to Wi-Fi, many location techniques can depend on external data for example the Network provider may need to communicate with Google’s servers to retrieve a location based on nearby Wi-Fi APs. Having mobile data on enables Wi-Fi (Network) based location even when the device is not connected to Wi-Fi. | ![]() |
Application permissions
Any application making use of location will need to request one or more permissions:
If the application is using the location manager or location services to determine the position of the device it will need to request one of the following:
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_COURSE_LOCATION" />
In addition, applications should also request the following feature if they are targeting Lollipop or higher:
<uses-feature android:name="android.hardware.location.gps" />
More information on the above settings can be found in the Android developer docs. Note that from Android Marshmallow onwards, considerations should be taken around runtime permissions where an application should request permission from the user via a dialog unless special steps are taken for Enterprise applications.
Activity recognition, either through the Location Services or via the Awareness API will require the following permission:
<uses-permission android:name="com.google.android.gms.permission.ACTIVITY_RECOGNITION " />
Applications making use of the Awareness API (which is backed by Google’s cloud services) will need to include an API key as well as separate keys for places and beacons. This is detailed in the Android developer docs for the awareness API.
Any application wishing to make use of mocked locations will need the following permission:
<uses-permission android:name="android.permission.ACCESS_MOCK_LOCATION" />
As previously mentioned, if this MOCK_LOCATION permission is included in a Manifest.xml used for a release build, Android Studio will give a linting error to indicate this permission is designed for testing or debugging only.
Indoor Versus Outdoor
Many of the Android APIs discussed thus far are focused on outdoor use cases, when moving indoors there are a number of additional challenges:
- No GPS is available (at risk of stating the obvious)
- The device may not have a network connection or the connection may be unreliable
- The resolution required for many indoor use cases requires precise location e.g. the customer is by the apples or my lost device is in aisle 3, shelf 2.
- Maps of indoors locations may not exist and therefore require site surveys to create, a manually intensive effort.
- The required maps may update very frequently as store layouts change.
- Battery life of Bluetooth Low Energy (BLE) beacons is finite, adding to maintenance costs.
- BLE beacon locations are fixed and require expensive effort to move the physical beacons and their virtual location in the corresponding cloud solution.
Although indoor locationing has many challenges, it is also an area of intense innovation right now by both established companies (including Zebra) and start-ups:
- Solutions available on the market can provide automated indoor mapping without the need for expensive site surveys, this might be done by some deep learning analysis of how signals scatter within the building or by drone.
- The established concept of BLE is being challenged by various companies, generating virtual beacons or marrying BLE with some bespoke proprietary technology to enhance location.
- For some time now Google (& Apple) have used the concept of ‘find my device’ where although a precise location cannot be known, a rough location can be given and the device then be further located by making it ring.
- As mentioned in the introduction, Zebra have a number of indoor locationing solutions based on Wi-Fi and RFID designed to meet a variety of customer needs.
This is a rapidly changing field and no single solution will suit every customer and use-case, one of the primary reasons Zebra produces so many different hardware form factors with different device capabilities is to provide as much choice as possible. As we move forward, although our devices all support Bluetooth we will see increased support for Lollipop which is the first Android version where Google considered their BLE implementation to be mature and so BLE becomes usable for more of our customers and partners.
Bluetooth Low Energy
The primary go-to technology for indoor locationing is Bluetooth Low Energy (BLE). Google have a fully functional BLE API but a device detecting nearby beacons is only a part of the overall solution, there needs to be a server-side component to associate those beacons with a physical location and in most cases perform some dynamic action. The customer does not care that they are stood by the apples (they have eyes to see that), they are more concerned that because they have not purchased apples in 2 weeks they are eligible for a special 10% off coupon.
I mentioned Google’s Beacon platform when discussing the Awareness API but there are many other similar platforms that exist from other companies. At the time of writing, Zebra does not recommend any specific beacon platform and our Bluetooth hardware is compliant with Bluetooth standards so will support any platform of the customer’s choosing.
Enterprise Considerations for Non-GMS devices
Many enterprises choose to opt for Non-GMS devices for a variety of reasons but doing so limits your NETWORK_PROVIDER to providing cellular only based locations. One option for non-GMS devices is to use the Google Geolocation REST API which takes a series of Wi-Fi access points and returns a best guess of the device lat / long. By scanning for nearby APs using the WifiManager and then passing the found APs to the REST API you get a fairly good approximation of the network provider functionality offered by GMS devices. This technique does of course involve passing data through Google’s servers and acquiring an API key which depending on usage may be a paid service to Google. Your application will also need to request the ACCESS_WIFI_STATE permission.
Sample Application
It seems to be a theme whenever I write a blog, there is always a sample application to go along with it and this blog is no exception.
The application is available on github at https://github.com/darryncampbell/Location-API-Exerciser, is released under an MIT license and whilst documentation is scarce the UI is hopefully self-explanatory. I have tested on a Nexus 6P N, TC51 M GMS and TC55 KK GMS. My colleagues have also tested on a TC75 KK as well as their personal phones.
The application offers the following functionality:
- Whether or not Location is enabled (via the setting) and whether or not the location services are available (accepted in the start-up wizard)
- The status of the various providers on the device available through LocationManager and LocationServices.
- The lat / long positions returned by the Network and GPS providers
- The lat / long position returned by the fused provider
- The ability to scan for nearby Wi-Fi APs and use Google’s Maps API to resolve those APs to a lat / long
- Buttons to exercise the location services SettingsApi to ensure device settings are correct (& prompt the user to automatically correct them)
- Output from activity recognition
- Ability to declare a geofence with both the location manager and location services APIs
- Output from reverse-geocoding from the various returned lat / long coordinates
- Exercise the Awareness API’s snapshot functionality (fences are not implemented)
- The ability to initiate a custom provider which will use the location manager API to send mocked data to the selected provider name. Requires the developer option to be set to use mock locations as explained earlier in this blog. The custom provider lat / long is reported towards the top of the UI.
I am being very trusting and including my application Awareness API keys in the manifest of the application for ease of use; they are not associated with a paid account so if the number of requests exceeds some amount (2500?) a day then you may see the Awareness functionality fail.
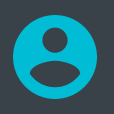
Darryn Campbell
1 Replies
Thanks Darryn.
From a recent Help Desk case, it was found that what at first glance is an Unexpected permission reason for Bluetooth BLE:
But for using the "BluetoothLeScanner" API without a filter, the need for Location Permissions is required due to the type of APIS that Google leverages under-the-covers for BLE use.
<a href="https://developer.android.com/guide/topics/connectivity/bluetooth-le">B… low energy overview | Android Developers</a>
-sean