PDF Printing
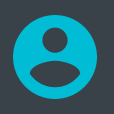
Anonymous (not verified)
48 Replies
Hi Mirco,
I haven't tried it on Android yet, but the way the PDF Virtual device works, you should be able to just send your PDF as a file. Try something like this code (using the Android SDK)
<code>import com.zebra.sdk.comm.Connection; </code>
<code>import com.zebra.sdk.comm.ConnectionException; </code>
<code>import com.zebra.sdk.comm.TcpConnection; </code>
<code>import com.zebra.sdk.device.ZebraIllegalArgumentException; </code>
<code>import com.zebra.sdk.printer.ZebraPrinter; </code>
<code>import com.zebra.sdk.printer.ZebraPrinterFactory; </code>
<code>import com.zebra.sdk.printer.ZebraPrinterLanguageUnknownException; </code>
<code>public class SendPDFExample { </code>
<code> public static void main(String[] args) throws Exception { </code>
<code> Connection connection = new TcpConnection("192.168.1.100", TcpConnection.DEFAULT_ZPL_TCP_PORT); </code>
<code> try { </code>
<code> connection.open(); </code>
<code> ZebraPrinter printer = ZebraPrinterFactory.getInstance(connection);</code>
<code> printer.sendFileContents("/sdcard/documents/sample.pdf"); </code>
<code> } catch (ConnectionException e) { </code>
<code> e.printStackTrace(); </code>
<code> } catch (ZebraPrinterLanguageUnknownException e) {</code>
<code> e.printStackTrace();</code>
<code> } catch (ZebraIllegalArgumentException e) {</code>
<code> e.printStackTrace();</code>
<code> } finally {</code>
<code> connection.close();</code>
<code> }</code>
<code></code> }
}
Hi Robin,
thanks for your post. I have one question: We have a QLN 220 Printer with Link-OS PDF Virtual Device but we don't know how to send PDF Files to the printer. We can't find any example or usefull SDK Api documentation. We use the native Android SDK. Can you tell we in detail how we have to send a PDF File?
Thanks in advance,
Mirco
Hi Robin,
thank you very much. It's easy and working. Sometimes we can't see the wood for the trees
Hi Hector, From the developer standpoint it's the same as sending any file.
#import "TcpPrinterConnection.h"
#import
#import "ZebraPrinter.h"
#import "ZebraPrinterFactory.h"
<a href="http://techdocs.zebra.com/link-os/latest/ios/content/interface_tcp_prin…; *zebraPrinterConnection = [[<a href="http://techdocs.zebra.com/link-os/latest/ios/content/interface_tcp_prin…; alloc] initWithAddress:@"192.168.1.101" andWithPort:6101];
BOOL success = [zebraPrinterConnection open];
NSError *error = nil;
id printer = [<a href="http://techdocs.zebra.com/link-os/latest/ios/content/interface_zebra_pr…; getInstance:zebraPrinterConnection error:&error];
// Send file to the printer
BOOL sent = [[printer getFileUtil] sendFileContents:@"/Documents/sample.pdf" error:&error];
if (error != nil || printer == nil || success == NO || sent == NO) {
UIAlertView *errorAlert = [[UIAlertView alloc] initWithTitle:@"Error" message:[error localizedDescription] delegate:nil cancelButtonTitle:@"Ok" otherButtonTitles:nil];
[errorAlert show];
[errorAlert release];
}
[zebraPrinterConnection close];
[zebraPrinterConnection release];
Hi Timo,
What you have should work fine. Are you getting an error?
The PDF printing is not part of any SDK, but an application you load on the printer to handle PDF documents directly. <a href="https://www.zebra.com/us/en/products/software/barcode-printers/link-os/… Direct - Virtual Device | Zebra</a>
Robin
Hi Timo,
On most OS's there are native APIs for just opening a standard TCP socket, opening and reading a file, and sending it to the socket. <a href="https://www.codeproject.com/articles/32633/sending-files-using-tcp">Sen… Files using TCP - CodeProject</a> . The default ports (depending on the printer) are 6101 and 9100.
If you want to use a standard printer without the virtual device, you have to convert the PDF on the app side. You can convert it to some image types that the printer will take directly. Most PDF converter libraries we've looked at are either expensive or not very good. iOS is the only OS we've looked at that has a decent native PDF converter.
Hope this helps,
Robin
Besides an installation manual (how to get the virtual device to the printer) I had no other documentation. I have the SDK you mentioned, but it doesn't mention anything on the PDF Virtual Device. But no worries, the functionality I wanted has been achieved, I can print PDF on my Zebra Printer from my program .
Do you have any infos on how I could do it without you API? Not that I want to change my programm, more out of technical curiosity.
Thanks for the support.
Hi, could you help me with the error below?
com.zebra.sdk.comm.ConnectionException: Error writing to connection: ZDesigner ZM600 200 dpi (ZPL).
The error occurs in the line below:
ZebraPrinter printer = ZebraPrinterFactory.getInstance(connection);
Robin, Unfortunately, not true. When trying to print it gives the printer a hint that there is something you want to print. But printing is not done. Note that the printer used is IMZ320. And through Bluetooth
I want to help . Thanks
Robin, Unfortunately, not true. When trying to print it gives the printer a hint that there is something you want to print. But printing is not done. Note that the printer used is IMZ320. And through Bluetooth
I want to help . Thanks
Hello John,
the orientation in SAP is part of the Adobe livecycle designer. The orientation depends on the width/length of the label and is set automatically. You can't change it manually.
I tried it with "Form calc" instructions in the XML, but I couldn't change the orientation in SAP..
Our current solution works like this: Landscape in Adobe > portrait orientation in the windows driver >> landscape output on ZEBRA
Landscape in Adobe > landscape orintation in win driver >> portrait output on ZEBRA (rotation 90°)
and so on.
Hans
Hi Hans,
Here is the URL for the Zebra Windows Drivers.
<a href="https://www.zebra.com/apps/dlmanager?dlp=-227178c9720c025483893483886ea…;
John
Hi Hans,
Zebra has only created device types to handle the SAPScript Upload method where the ZPL is wrapped around SAPScript. This solution is tied to the Zebra Designer for MySAP Business Suite or other tools designed to output ZPL, including manual coded ZPL. These device types are:
<strong>For SAP Release 4.6C and greater.</strong>
<strong>ZLB_ZEB.PRI</strong>, S9162.CPA (for Scalable fonts - IBM™ CP850)
<strong>ZLB_ZEBU.PRI</strong> (for Andale and Swiss 721 fonts - Unicode UTF-8)
The other device types created by Zebra support Smart Forms. These are the ZLZEB and YZB named device types. The IFbA device types are created by Adobe. Zebra has no device types that work with this solution at this point.
________________________________________________________________________________
The <strong>PDF Direct</strong> solution is available through your reseller or purchasing from the Zebra website. The URL below is where the <em>license can be purchased</em>.
<a href="https://www.zebra.com/us/en/products/software/barcode-printers/link-os/…;
Please note that the PDF Direct solution is only available on LinkOS printers. You should be able to use any device type that outputs a PDF, and then route that to the printer directly.
<em>PDF Direct works with LinkOS printers regardless of they are mobile or tabletop.</em>
________________________________________________________________________________
I think that if you use the SAPWIN driver, and you have a windows printer, you should easily be able to load the Zebra Windows Driver and route the job to the printer.
This is an example of how that process would work:
Print Job in SAP >>>
Routes through SAPWIN device type >>>
SAPWIN sends image to WIN Print Server >>>
WIN Print Server routes through Zebra Win Driver >>>
Zebra Win Drivers sends to printer / Prints label
This solution should work unless you decide to move away from having a Windows Print Server managing the print solutions.
________________________________________________________________________________
If you need additional information, please send an email to <a href="mailto:sapinfo@zebra.com">sapinfo@zebra.com</a> and <a href="mailto:jramsey@zebra.com">jramsey@zebra.com</a>
Hi Hans,
The process for PDF Direct would be:
Print Job in SAP as <strong>PDF</strong> >>>
Routes through <strong>SAPWIN device type (maybe another PDF Device type) </strong> >>>
Win Spooler sends to <strong>Zebra printer ZT410 with PDF direct</strong> / Prints label
There is no need to pass the PDF to a windows driver. If you still want to use the Windows Printer Spooler/Server, you can do so but will need to pass the PDF through a raw or generic text driver. The reason for this is you do not want anything to be added to the print output. The Zebra Windows driver takes the image it receives, converts it to one that the printer understands, and then passes it to the printer.
Best Regards,
John
Hello John,
Thanks for the answer. I will test it as an alternative to our current solution.
There's stiil a question in my mind. How can I turn the output by 90° without a driver ?
All our labels are designed in format landscape, e.g. 4x2inch, 6x4 inch.......
Today we turn the label in the windows driver by 90° and print portrait format with max. 4 inch width.
How can I turn the output without an driver ? In the printer configuration there's no parameters to turn the output.
Hans
Hi Hans,
Rotation can only be performed by a Windows Driver after it is sent from SAP. The reason for this is that the output file is coming down as an image or ZPL with a specific orientation. The windows driver can make the rotation modification on the fly. If ZPL is the output then it is hardcoded with a specific rotation. The only way to change the rotation in ZPL is to modify it with the correct rotation ZPL.
Is there a reason why you are not rotating it to portrait directly from SAP?
John
Hi Hans,
This sounds like an issue within Adobe Livecycle Designer. Let me ping Adobe to see if there is a solution for rotation. They sometimes take a long time to respond, but hopefully they will have a solution.
John
Hello John,
you proposed a raw or generic text driver. I saw that there's a parameter for label orientation !!!
Thanks for your support.
Hans
Hi Hans,
The raw or generic driver will not change the orientation (when sending ZPL) as it is designed as a passthrough. I am not sure what the results would be if you were sending a PDF. It may rotate a PDF, so my suggestion would be to try it and let me know the results.
The problem arises when you send a PDF to the printer with the PDF direct solution. The reason for this is that the PDF has its rotation already set within its code. I am not sure if a raw/generic driver can actually manipulate the PDFcode set. Again, this is one of those things that is best tested to see the results.
John
Hello Steve,
I wrote my question wrong, i meant. which printer driver do you use on the print server?
Hans
Hello John,
thanks for your suggestion. In the past I tested different printer types in SAP, the AZPL-drivers, the Zdesigner-driver on the print server.
But at the end I hope to find a Zebra supported solution.
We are using the IFbA only for print output. Adobe livecycle designer is our first choice for all forms, also for bigger forms for thermal printers (min. 1x2inch). For mini forms like part labels (e.g. 0.5x1.5inch) we use the printer programming language.
With the PDF format we are independant of the printer. Laser printers are no problem, but thermal printers. We can't use only 1 brand, because the technical support worldwide is very different. So we have 3 different brands.
I am very interested in the PDF direct. Could you give me concrete instructions how to realize it ? Which SAP printer type, SAP protocol type, do we need SAPsprint, which driver on the print server ?
In 2018 we will start with mobil printers e.g. QLN420 like Steve told it. Will PDF direct work with it ?
I would be grateful for any advice
Hans
Hi John,
yes I will test it and I'll tell you the results. I have to talk with our dealer to get a test "PDF print".
It will last, and first it is christmas.
best regards, merry christmas and a happy new year
Hans
Thank you a lot, Robin The guide on <a href="http://www.rasteredge.com/how-to/csharp-imaging/pdf-creating/">PDF printing</a> is of great help to my work. Best regards.
Hi Timo,
The .NET SDK dll is in the <a href="https://www.zebra.com/us/en/products/software/barcode-printers/link-os/… Multiplatform SDK | Zebra</a> download.
You can send the file a number of ways, but if you are using a Windows Mobile/CE device, this is probably easiest. You can send the file to the printer over TCP, Bluetooth, USB, or nearly any other communication method supported by your printer. You don't have to use our SDK, but we have built in some API's to make it easier. What kind of documentation are you looking for?
Robin
Update: for windows .NET:
-link ZSDK_API.dll to project
ZebraPrinterConnection zc = new TcpPrinterConnection("192.168.100.111", TcpPrinterConnection.DEFAULT_CPCL_TCP_PORT);
zc.Open();
ZebraPrinter zp = ZebraPrinterFactory.GetInstance(zc);
FileUtil fu = zp.GetFileUtil();
fu.SendFileContents(@"C:\SomeFile.pdf");
zc.Close();
Is there any documentation on this?
Is there a version for .NET printing of PDF to the Virtual Device?
No, it works fine, I just needed the DLL from the SDK to send the PDF to the Virtual Device Equipped printer. Or is there any other way to send the file? I don't have any documentation on the Virtual Device sadly (as I cannot find any)
Hi,
I need to print PDF generated from a web app (using SSRS and export to PDF) to a S4M printer, but unfortunately I cannot find PDF Virtual Device for this printer.
Can you point me where to find this device?
Otherwise, what other options I have to be able to print labels from a web application.
The web application is hosted in cloud and the users are accessing it over internet.
While the S4M printer is local (either connected through USB or as network printer).
Thank you
Zebra does offer a PDF Virtual Device for Link-OS printers: <a href="https://www.zebra.com/us/en/products/software/barcode-printers/link-os/… Direct - Virtual Device | Zebra</a>
Hi,
We have a QLN420 with Link OS and PDF license.We are trying to print from SAP but nothing is coming out. It triggers the printer but it just feeds blank pages.
Do we setup the device as a PDF printer in SAP and send the spool to it or are we supposed to send the file direct to it via tcp ?
Hi Daniel, i don't understand if is necessary to buy PDF Virtual Device for print any pdf document in Xamarin.Forms project [for Ios and Android] or if is not necessary (and if is possible by blutooth connection).
Thanks in Advance,
Nicola
Hello Steve,
we use SAP and a lot of ZEBRA printers in our plants in US and have the same problem/Questions similar to yours.
Did you ever got an answer or figured it out yourself ?
Thanks in advance
Hans
I never got a reply that made much sense from Zebra in terms of ABAP/Config.
The Direct PDF add-on for the printer requires that the PDF in base64 to be sent direct to the printer via a TCP port
That can’t be easily done in ABAP, so why they are saying it’s a solution for SAP in a bit confusing.
What you can do, and how we resolved it, was to setup the mobile printers on a print server, not direct from SAP.
When connected directly to a PC, you can send a PDF to it without doing anything special because it has a PCL driver and windows does the rest.
Do not use the ZPL driver, use the PCL driver on the print server.
Setup the printer in SAP as a PDF1 with access type U
Then call FM ADS_CREATE_PDF_SPOOLJOB with the base64 as an xstring.
This does not need the PDF Direct add-on.
Thanks for your quick reply. I have had similar experiences as you.Your solution with the ADS-function is very interesting, because next in 2018 we start also with mobile label printers.Our labels are Adobe forms in SAP. The PDF of the ADS is sent to ZT410 printers defined as device type PDF1 with Berkeley protocol. On the Windows print-servers the SAPsprint/SAPPDFprint-Services run. The Zebra printers are connected to the print-servers. But we use the zebra printer driver from Bartender (Seagull). Depending on the orientation, landscape or portrait and the label dimensions must be set. It works, but unfortunately it is not very flexible.
My question: when I would test the ADS-function, which driver should I take for the ZEBRA printer ?
thanksHans
That function module only works for SAP printers that have type PDF1
Hi Hans,
In Adobe Forms, you should have the capability to utilize the device types that were created by Adobe, for the Zebra printers. If I recall correctly, the device types that were created by Adobe are named AZPL200, AZPL300, and AZPL600, with the last 3 numbers referring to the DPI (dots per inch) of the printer. The device types are used to output the native Zebra programming language called ZPL. In an ideal Zebra printing application, the printer receives ZPL. This enables faster processing printer side, better quality barcodes, and lowers the amount of network traffic as the file is more often smaller than when sending an image.
Some customers decide that the PDF is their ideal output. This approach requires that the PDF routes through a driver and converts the PDF to an image that the printer understands. You mentioned that you are using the Seagull driver. You can also try using the Zebra Printer drivers (available on the Zebra website) designed to work on Windows. Zebra also recently introduced a solution called "PDF Direct". The allows a PDF to bypass a windows driver and be processed directly in the printer. This solution requires a purchased license per printer.
My suggestion is that you try to use the IFbA device types. If you are using the interactive portion of IFbA, then we may need to modify the approach to your printing solution. If this is the case, please comment so we can discuss further.
Best Regards,
John Ramsey
Hi Hans,
Maybe your local reseller will have a printer with Direct Print loaded on it so that you can test it before you purchase a license. If you desire the PDF Direct license immediately, you can purchase it online from Zebra.
<a href="https://www.zebra.com/us/en/products/software/barcode-printers/link-os/… Direct - Virtual Device | Zebra</a>
Merry Christmas and a Happy New Year to you and yours as well!
John
Hello John,
thanks for your suggestion. In the past I tested different printer types in SAP, the AZPL-drivers, the Zdesigner-driver on the print server.
But at the end I hope to find a Zebra supported solution.
We are using the IFbA only for print output. Adobe livecycle designer is our first choice for all forms, also for bigger forms for thermal printers (min. 1x2inch). For mini forms like part labels (e.g. 0.5x1.5inch) we use the printer programming language.
With the PDF format we are independant of the printer. Laser printers are no problem, but thermal printers. We can't use only 1 brand, because the technical support worldwide is very different. So we have 3 different brands.
I am very interested in the PDF direct. Could you give me concrete instructions how to realize it ? Which SAP printer type, SAP protocol type, do we need SAPsprint, which driver on the print server ?
In 2018 we will start with mobil printers e.g. QLN420 like Steve told it. Will PDF direct work with it ?
I would be grateful for any advice
Hans
Hi John,
thanks for your detailed explanation. I miss the PDF direkt.
Does this process description - nearly the same like yours - will work with PDF direct ?
Print Job in SAP as <strong>PDF</strong> >>>
Routes through <strong>SAPWIN device type</strong> >>>
SAPWIN sends image to WIN Print Server (<strong>SApsprint/SAPPDFprint</strong>) >>>
WIN Print Server routes through <strong>Zebra Win Driver (Zdesigner driver)</strong> >>>
Zebra Win Drivers sends to <strong>Zebra printer ZT410 with PDF direkt</strong> / Prints label ?
Is there any file size or any other restriction while printing PDF files through PDF Direct?
Actually I have printed few PDF files through PDF Direct in the ZD620d printer, some of them not printed, it prints as blank.
Please share your thoughts and suggestions to resolve this issue.
Hi John
I am trying to print PDF from Enterprise browser. ZPL works fine , but I cannot find any way in docs to print PDF requested from Api to Mobile printers. Our printers are installed with PDF licenses and they print PDF from Pc Browsers perfectly fine. Can you please provide some information on this