The last (Javascript) frontier: RhoConnect source adapters. Part 1.
By now, if you have followed the Launchpad forums or blogs at all, you already know that Javascript has received a lot of attention in RhoMobile Suite 4.0 and with full access to every API at the same level as Ruby, writing enterprise mobile apps completely in Javascript is now possible.
If that was not good enough, RhoMobile 4 has another pleasant surprise for you: RhoConnect, the server that handles two-way synchronization between a RhoMobile app and an enteprise data store, can now be programmed in Javascript too. Javascript programmers of the world, rejoice in the knowledge that your favorite language can now take you all the way from the client to the server and back. Using only Javascript, you can now write the server-side part of a RhoMobile application too, thanks to the new built-in RhoConnect-Node.js integration.
Getting started with Javascript in RhoConnect
Assuming you have installed RhoMobile Suite 4.0 and Node.js already, the first step to start working with RhoConnect and Javascript together is to generate a new Javascript-enabled RhoConnect application:
rhoconnect app testserver --js
next, add your first source adapter: Product
cd testserver
rhoconnect source Product --js
That command will create models/js/product.js with code similar to the following:
var rc = require('rhoconnect_helpers');
var Product = function(){
this.login = function(resp){
// TODO: Login to your data source here if necessary
resp.send(true);
};
this.query = function(resp){
var result = {};
// TODO: Query your backend data source and assign the records
// to a nested hash structure. Then return your result.
// For example:
//
// {
// "1": {"name": "Acme", "industry": "Electronics"},
// "2": {"name": "Best", "industry": "Software"}
// }
resp.send(result);
};
this.create = function(resp){
// TODO: Create a new record in your backend data source. Then
// return the result.
resp.send('someId');
};
this.update = function(resp){
// TODO: Update an existing record in your backend data source.
// Then return the result.
resp.send(true);
};
this.del = function(resp){
// TODO: Delete an existing record in your backend data source
// if applicable. Be sure to have a hash key and value for
// "object" and return the result.
resp.send(true);
};
this.logoff = function(resp){
// TODO: Logout from the data source if necessary.
resp.send(true);
};
this.storeBlob = function(resp){
// TODO: Handle post requests for blobs here.
// Reference the blob object's path with resp.params.path.
new rc.Exception(
resp, "Please provide some code to handle blobs if you are using them."
);
};
};
module.exports = new Product();
If you have ever developed RhoConnect source adapters in Ruby, you will immediately recognize the structure and methods there. If this is your first time, fear not: writing RhoConnect source adapters is one of the most rewarding coding activities you can find in terms of the results you get for the small effort required and the rest of this article will walk you through the process and, in the second part of this series, we will actually build one from scratch.
A RhoConnect source adapter does two things:
- connect to a backend data source (SQL, flat file, REST API, anything) and return data to a mobile application
- receive data from a mobile application and upload it to the backend
It is the bridge that connects your RhoConnect server (and hence your RhoMobile app) to any existing data source.
The RhoConnect server does most of the heavy work for you and your task is reduced to just implementing the methods in the adapter:
query
The query method is equivalent to the "select" operation in a database. It is called to fetch data from the backend and return it to a RhoMobile app. The value you return must be a hash, with keys representing identifiers and the values being another hash representing an object. Let that sink in for a second and see the following example:
this.query = function(resp){
var result = {
"1": {"name": "ET1", brand: "Motorola", "sku": "12345"},
"2": {"name": "iPhone 5C", brand: "Apple", "sku": "67890"}
}
resp.send(result);
};
As you can see, this very simple adapter will return two hardcoded products. There is no need for the keys to be numbers and in fact, they are actually strings and not numbers (note the quotes). The following example using GUIDs will have the same result:
this.query = function(resp){
var result = {
"6e13b279-182d-4922-ace0-6672cd4dd63d": {"name": "ET1", brand: "Motorola", "sku": "12345"},
"14014408-fcce-48a8-8171-c6bd321e7d7b": {"name": "iPhone 5C", brand: "Apple", "sku": "67890"}
}
resp.send(result);
};
These identifiers become the link between objects on a RhoMobile application and the RhoConnect server.
create
When a RhoMobile application creates a new object, it will be initially stored in the local database of the device. Once a synchronization takes place, the create method in the javascript source adapter will be called for you to insert the data into the backend as necessary. The data for the new object you are expected to create is in a hash called resp.params.create_object.
Similarly to "query", you must send as a return the identifier of the item that was just created but this time just a string value. There is no hash to be returned, since create only deals with one object at a time.
update
Once a RhoMobile application can synchronize data with a RhoConnect server, you will usually want to make changes to that data and send updates back to the server. The update method does just that: receive changes from the mobile app and apply them to the backend.
The data for the new object you are expected to create is in a hash called resp.params.update_object. That hash will not contain every property of the object, just those that were changed in the mobile app.
del
As its name implies, del (shorthand for delete) is the method in charge of deleting objects from the backend when they are deleted in a mobile app.
Apart from those CRUD methods, there is also login and logoff, which are called at the beginning and end of the synchronization process, and allow you to, for example, establish a database connection.
As you can see, following this very simple structure you can start adding two-way synchronization capabilities to your application in no time.
Now is a great time to start experimenting with Javascript source adapters, in anticipation of the public RhoMobile 4 release. See you in part 2 of this series, where we will build a complete source adapter from scratch completely in Javascript and see how to interact with a database.
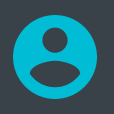
Kutir Mobility
Replies