How to prevent Multiple Instances of Applications in Windows Mobile
Over the last several years most partners have had multiple instances of their application running on the device which has
caused some issues for them. It has been my experience that the partners need to check for this in their apps. There are several ways to check for this
depending on what programming language is used. Here are some links and snippets of code:
int _tmain(int argc, _TCHAR* argv[])
{
// CREATE A SINGLE INSTANCE MUTEX
HANDLE hMutex = CreateMutex(NULL, FALSE, L"ApplicationNameSingleInstanceMutex");
if (hMutex != NULL)
{
if (GetLastError() == ERROR_ALREADY_EXISTS)
{
// MUTEX ALREADY EXISTS, EXIT APPLICATION
CloseHandle(hMutex);
return -2;
}
}
else
{
// ERROR CREATING MUTEX
return -1;
}
// NORMAL APPLICATION PROCESSING HERE
// CLOSE HANDLE TO MUTEX
CloseHandle(hMutex);
return 0;
}
Also please check this post out :
I have included another link here:
http://www.nesser.org/blog/archives/56
and a copy of his code: The below was needed because users were not patient : Most users are not. Here is some sample code: This is for C#
using
System;
using
System.Windows.Forms;
using
System.Runtime.InteropServices;
namespace
SomeNameSpace
{
static
class
SingleInstance
{
[MTAThread]
static
void
Main()
{
// Check if we have a duplicate
instance of the program running
if
(
IsInstanceRunning() )
{
// Perhaps log the fact a duplicate
program was shut down
return;
}
/*
* Continue with your normal program here
*/
try
{
Application.Run( YourFormClass );
}
catch
( Exception
e )
{
// Log an exception that caused the
application to close here
MessageBox.Show( "See log file
for details.\r\nClosing this application.", "Fatal Application
Error");
}
}
#region OpenNETCF native interface
to mutex generation (version 1.4 of the SDF)
public
const
Int32
NATIVE_ERROR_ALREADY_EXISTS = 183;
#region P/Invoke Commands for
Mutexes
[DllImport(
"coredll.dll", EntryPoint="CreateMutex", SetLastError=true)]
public
static
extern
IntPtr
CreateMutex(
IntPtr lpMutexAttributes,
bool
InitialOwner,
string
MutexName
);
[DllImport(
"coredll.dll", EntryPoint="ReleaseMutex", SetLastError=true)]
public
static
extern
bool
ReleaseMutex(
IntPtr hMutex );
#endregion
public
static
bool
IsInstanceRunning()
{
IntPtr hMutex = CreateMutex(
IntPtr.Zero, true, "ApplicationName");
if
( hMutex == IntPtr.Zero )
throw
new
ApplicationException(
"Failure creating mutex: "
+
Marshal.GetLastWin32Error().ToString( "X") );
if
(
Marshal.GetLastWin32Error() == NATIVE_ERROR_ALREADY_EXISTS )
return
true;
else
return
false;
}
#endregion
}
}
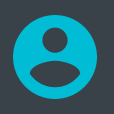
Randolph Kaiser
1 Replies
Usually, Windows Mobile will keep only one instance of the application, but this is true only after the first window has been created.
If you have a lot of code to do, or time consuming tasks like opening DB, before the creation of the first windows, then, the OS will allow another instance of the same App. On my testing, I've been able to create more than 20 instances of the same application just tapping the icon fast.
There are several ways to avoid that, one is what you mention here and another, could be using ATOM variables to keep only one instance. So your code could be like this:
#define ATOM_MY_APP L"AtomMyApp"
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPTSTR lpCmdLine, int nCmdShow)
{
if(GlobalFindAtom(ATOM_MY_APP))
return 0;
ATOM atomApp = GlobalAddAtom(ATOM_MY_APP);
//
// Your code goes here
//
GlobalDeleteAtom(atomApp);
return 0;
}