Alternatives to GMS Mapping
Introduction
Mobile devices today are a central hub for computing services. Unlike the cell phones of old that could only perform one function, modern devices are expected to be Swiss Army knives, multi-talented and connected to the wider cloud of Internet services.
Unlike consumer Android devices, Motorola Solutions’ enterprise devices do not have Google Mobile Services (GMS) installed. GMS provides such applications as Google Marketplace, Google Mail, and Google Maps, as well as many other Google features and services that are standard on stock Android devices.
The primary reason why these services aren’t installed is that any device that has GMS installed has its data routed through Google’s network which Google, of course, could mine for information. Many enterprises do not want their data accessed by third-parties and are therefore hesitant to allow such services on their company’s devices.
Since GMS is not available on Motorola Android devices, how do you develop and deploy mapping applications on Motorola’s Android devices like the ET1, TC55, or MC40? In this article we’ll discuss what options are available to developers when it comes to providing mapping solutions on Motorola hardware.
What are Location Services?
Many developers of enterprise-level mobile applications have to provide a solution for not only their core product functionality, but also ancillary services that support and extend that functionality. One of the most important of these services are location services.
Location services can be broken into a variety of categories:
- Geolocation - translating your current location to latitude and longitude values.
- Mapping - taking a set of coordinates and displaying a map depicting that location with various features.
- Routing - using two or more locations to create a path that intersects all points
- Route Optimization - calculating an optimal path between locations that meets a set of criteria (shortest distance, fastest time, etc.).
- Turn-by-Turn Navigation - using an optimized route to generate a set of instructions that can be followed real-time to each destination.
A mobile application may need to integrate one or more of these types of services into its workflow. For example, an application might need to display a map of the location of a job, or may need to provide turn by turn directions from the current location to the next customer. These needs can be answered by adding location services to your application.
Internet Connection Needed
While there are several options for mapping and location services, most of them require access to the Internet in order to access APIs provide the necessary data for use. Also, in order to acquire the current location of the device automatically GPS services must be available and activated. The combination of real-time GPS and Internet connectivity provides access to the greatest set of location service features.
RhoMobile Location Services: MapView
The RhoMobile platform provides basic mapping APIs using the MapView class (http://docs.rhomobile.com/en/4.1.0/guide/maps). The MapView can provide basic services such as geolocation and mapping, but it does not provide routing or navigation services.
MapView can work with different mapping providers, such as ESRI, Google, and Open Street Maps (http://www.openstreetmap.org). While these services are capable within their bounds be aware that they are not all uniformly available across platforms.
Native Mapping
Most mobile platforms provide native mapping services. These services can be tapped into when developing applications that will only target that specific set of devices, or are conditionally coded to do so.
Not all native mapping apps are available across all platforms. If your requirements are for a uniform mapping solution, using RhoMobile along with a third-party cross-platform mapping product is a viable solution.
Third-Party Mapping
Given that the native mapping solutions for RhoMobile can limit you feature-wise and have limited cross-platform support, there is a rich market for third-party solutions to fill the gap.
The solutions fall into two primary categories:
- Services that use free mapping data
- Services that use proprietary mapping data
Open Street Map
The main difference between the free and proprietary solutions is the level of accuracy, the coverage of the mapping, and the maintenance of the data over time.
Open Street Map (OSM, http://en.wikipedia.org/wiki/OpenStreetMap) is perhaps the most popular of the free solutions. OSM gathers its mapping data from government sources and other public domain databases and then relies on crowd-sourcing to maintain the data. OSM mapping data is available as an Open Source project.
While OSM data accuracy and coverage is improving each day it is not as complete as commercial products. Still, depending on the level of accuracy your application requires it can be an inexpensive and competent solution.
Commercial Services
There are a number of large, mature mapping solutions that provide access to their data via APIs. These services offer more than just simple mapping, but offer a complete set location services.
- MapQuest mapping (http://www.mapquestapi.com)
- Bing Maps (http://msdn.microsoft.com/en-us/library/ff701713.aspx)
- ESRI (https://developers.arcgis.com/en/)
The cost of these services vary depending on what features you wish to access. These providers also have a “Community” level of access to their APIs that provide access but with certain usage limitations. These community-level services are a great way to try out a provider’s offerings without having to make a financial commitment.
Client-Side Frameworks: Leaflet
Once you’ve chosen a service provider you still need a method to display the mapping, routing, and navigation data in the client. While most commercial services will offer their own proprietary solution there is another choice: Leaflet (http://leafletjs.com).
Leaflet is a “An Open-Source JavaScript Library for Mobile-Friendly Interactive Maps”. It provides an agnostic interface for displaying map and route data within your mobile application.
Leaflet’s API is easy to use and consistent, regardless of what provider you pair it with.
For the purpose of demonstration I’m going to use MapQuest’s API to show off Leaflet’s capabilities.
MapQuest API
MapQuest (http://www.mapquestapi.com) offers a full set of location-based services, from geocoding to turn-by-turn navigation. The services include:
- Data Manager Service
- Directions Service
- Geocoding Service
- Long URL Service
- Search Service
- Static Maps Service
- Traffic Service
- Route Planner
MapQuest provides open source mapping solutions using Open Street Map, as well as commercial services using their proprietary mapping databases. Both services can be accessed using the Leaflet API.
You can sign up for access to the services by creating a MapQuest API account at http://developer.mapquest.com. The account provides you with an API key that you can use to access the service. You will include this API key in all of your calls to the data provider.
A Basic MapQuest Example
To add it to your application you add the following to your page’s tag:
Note that the code above includes files implemented by the Leaflet team as well as MapQuest-specific files for accessing resources. The latter require that you provide your MapQuest API key for access.
Also, in the you provide some Javascript that gets called on page load:
type="text/javascript">
window.onload = function() {
L.map('map', {
layers: MQ.mapLayer(),
center: [ 37.785834, -122.406417 ],
zoom: 10
});
};
This code creates the map that will be inserted in a div with the id of “map”. The center
is the latitude and longitude of the center point of the map. The zoom
provides the zoom factor of the initial view. Note that the main Leaflet object is named simply ‘L’. All functions are methods off of this object.
In your index.rb
all you need to do is to add the target div
:
id="map">
Once the page is rendered the div will be replaced with an interactive map centered on the provided coordinates. While in the above example the coordinates are hardcoded you can quite easily make this dynamic based on a user’s input.
Routing Service
Simple mapping is the most fundamental use of the service. The Leaflet API also allows you access to routing services from MapQuest. Add the following to your :
src="http://www.mapquestapi.com/sdk/leaflet/v1.0/mq-routing.js?key=PLACE_KEY_HERE"
type="text/javascript">
This will enable access to MapQuest’s routing API.
The Javascript to call the routing API takes a few more parameters:
window.onload =function(){
var map, dir;
map = L.map('map', {
layers: MQ.mapLayer(),
center: [ 38.895345, -77.030101 ],
zoom: 13
});
dir = MQ.routing.directions();
dir.route({
locations: [
{ latLng: { lat: 38.895211, lng: -77.036495 } },
{ street: '935 pennsylvania ave', city: 'washington', state: 'dc' }
]
});
map.addLayer(MQ.routing.routeLayer({
directions: dir,
fitBounds: true
}));
};
The first thing we do is create a map object:
map = L.map('map',{
layers: MQ.mapLayer(),
center: [ 38.895345, -77.030101 ],
zoom: 13
});
Again we call the map method to generate a map that will replace the ‘map’ div
on the page. We create a MapQuest map layer that will manage the map components and we center the map on the coordinates 38.895345, -77.030101.
Leaving it at this point would simply draw the map as we had done in our earlier example.
The next thing we do is create a MapQuest routing object using:
dir = MQ.routing.directions();
This object will handle communicating our desired route to MapQuest, as well as drawing the route on our local map.
With our newly created route object dir
we pass in the list of points that we want to display in our route:
dir.route({
locations: [
{ latLng: { lat: 38.895211, lng: -77.036495 } },
{ street: '935 pennsylvania ave', city: 'washington', state: 'dc' }
]
});
Notice that we can provide the stops in our routes in a variety of ways: as latitude/longitude pairs or street address information. If we pass in the address the routing service will automatically perform the lookup to convert the address to coordinates.
One thing to note, the routing service requires two locations in order to create a valid route. If you pass only one location it will generate an error.
Finally, we need to add the route to our map in it’s own layer:
map.addLayer(MQ.routing.routeLayer({ directions: dir, fitBounds: true}));
The fitBounds:
parameter forces the map to adjust it’s zoom so that it encompasses the entire route.
Again, while the above data is static you can very easily replace the static calls with data-bound values or even have the user enter the information themselves. The process remains the same no matter what the data source.
Conclusion
Location services are an important part of mobile applications today. Adding those services cross-platform can be a challenge given the wide variety of services each device may provide. One way to simplify this complexity is to layer a single solution across all of the platforms you wish to support.
One of the other benefits of using MapQuest, or the other solutions mentioned here, is that it is agnostic of the framework being used; meaning it is not tied to RhoMobile, or even limited to mobile devices. This allows you to easily develop applications on the platforms you wish to support without having to worry about the specifics of the mapping implementation.
Motorola GMS Support on the TC55
Recently, Motorola announced that the TC55 handheld would be available optionally with GMS installed (http://www.motorolasolutions.com/XU-EN/Business+Product+and+Services/Mobile+Computers/Handheld+Computers/TC55). This gives you the flexibility of choosing a more secure platform or, in the case of GMS, a more feature-rich environment.
Online Resources
This is but touching the surface of both the Leaflet and MapQuest APIs. I recommend you refer to their documentation to get a feel for what the service offerings provide. The MapQuest API site (http://www.mapquestapi.com) has several interactive examples that will provide a look at both the output and the calls to the API that generate them.
For more on RhoMobile’s built-in mapping features check out the Rhodes System API Sample App at https://github.com/rhomobile/rhodes-system-api-samples.
The code samples above are implemented in a RhoMobile app that shows off the functionality of the APIs in a mobile application. You can download a copy of the demo app at https://github.com/chronosafe/mapping.
About us
Facing challenges with your RhoMobile implementation? Kutir Technologies, a Motorola Certified partner, can help you get your app done on time. Get in touch with us today at info@kutirtech.com , no strings attached.
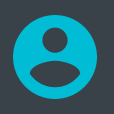
Kutir Mobility