Ionic Applications on Zebra Devices
Update (31st January 2019):
The Ionic Native Web-Intent package has recently had v5.0.0 released to the latest branch. This contains several fixes which means it is now possible to use WebIntent rather than accessing the 3rd party plugin discussed in this post directly. I have updated the sample application in a separate branch to show how to do this, GitHub - Zebra/ZebraIonicDemo at Transition_To_WebIntent since not all developers will be ready to transition to Ionic Native v5.0.0. To avoid confusion, the sample app's master branch remains unchanged for now.
Zebra has an active developer community that frequently gets questions about how to develop Ionic applications for our devices. About 10 years ago, it was perfectly acceptable for mobile apps aimed at industry to be utilitarian. But with the proliferation of smartphones, user expectations are now much greater, regardless of whether the app will be used by a handful of employees in a retail store or exist solely in the warehouse, the application needs to function well and look great. It is therefore no surprise that the Ionic Framework’s ability to rapidly develop beautiful-looking and robust applications with existing tooling is a great fit for Zebra devices.
Of course, Zebra offers a native SDK for Android, but unless you need extremely tight integration, I would not recommend developing a native Ionic plugin. Upwards of 95 percent of applications on Zebra devices are built to scan barcodes with the device’s dedicated scanning hardware. For these use cases there is a simpler solution for Ionic integration – DataWedge, a service resident on every Zebra device that supports an intent-based interface to control and interact with the device scanner without worrying about threads, timing or pending reads.
In this blog, I'll take you through how to create an Ionic application for a Zebra Android device capable of scanning barcodes and controlling the scanner configuration. The finished sample is available on the Zebra GitHub page at https://github.com/Zebra/ZebraIonicDemo.
The Android Intent Plugin
To interact with the DataWedge service to control the scanner we need to add an Ionic plugin capable of handling Android Intents. Ionic’s recommended web intent plugin will give us what we need, it is based on a Cordova plugin released under MIT license, so there are no issues including it in a commercial application:
Ionic Cordova plugin add com-darryncampbell-cordova-plugin-intent
The Code
With the Ionic application capable of sending and receiving Android intents, we can set up a broadcast receiver to handle both barcode scans and notifications from the DataWedge service.
(window).plugins.intentShim.registerBroadcastReceiver({
filterActions: [
'com.zebra.ionicdemo.ACTION',
'com.symbol.datawedge.api.RESULT_ACTION'
],
filterCategories: [
'android.intent.category.DEFAULT'
]},
function (intent) {
// Broadcast received
console.log('Received Intent: ' + JSON.stringify(intent.extras));
});
DataWedge needs to be configured to send intents to our broadcast receiver. DataWedge configuration is profile-based; profiles are associated with an application or activity, and when the specified app or activity comes to the foreground the associated profile configuration is applied. There are three steps to configure DataWedge:
- Create a DataWedge profile
- Associate the created profile with the Ionic application
- Configure the profile to send broadcast intents when a barcode is scanned
These three steps can be done manually or, if you are running a recent version of DataWedge on your device, can be done entirely in code.
The manual steps (with screenshots) are detailed in the sample project’s setup instructions, but it is far more convenient for both yourself and device administrators to configure the device in code:
1. Create a DataWedge profile
This is achieved with the CREATE_PROFILE API (requires DW 6.3+):
(window).plugins.intentShim.sendBroadcast({
action: 'com.symbol.datawedge.api.ACTION',
extras: {
"com.symbol.datawedge.api.CREATE_PROFILE": "ZebraIonicDemo"
}
},
function () { }, // Success in sending the intent, not success of DW to process the intent.
function () { } // Failure in sending the intent, not failure of DW to process the intent.
);
2. Associate the created profile with our Ionic application
This is achieved with the SET_CONFIG API (requires DW 6.4+):
let profileConfig = {
"PROFILE_NAME": "ZebraIonicDemo",
"PROFILE_ENABLED": "true",
"CONFIG_MODE": "UPDATE",
"PLUGIN_CONFIG": {
"PLUGIN_NAME": "BARCODE",
"RESET_CONFIG": "true",
"PARAM_LIST": {}
},
"APP_LIST": [{
"PACKAGE_NAME": "com.zebra.zebraionicdemo",
"ACTIVITY_LIST": ["*"]
}]
};
(window).plugins.intentShim.sendBroadcast({
action: 'com.symbol.datawedge.api.ACTION',
extras: {
"com.symbol.datawedge.api.SET_CONFIG": profileConfig,
"SEND_RESULT": this.requestResultCodes
}
},
function () { }, // Success in sending the intent, not success of DW to process the intent.
function () { } // Failure in sending the intent, not failure of DW to process the intent.
);
3. Configure the profile to send broadcast intents when a barcode is scanned.
Again, this is achieved with the SET_CONFIG API (requires DW6.4+):
let profileConfig2 = {
"PROFILE_NAME": "ZebraIonicDemo",
"PROFILE_ENABLED": "true",
"CONFIG_MODE": "UPDATE",
"PLUGIN_CONFIG": {
"PLUGIN_NAME": "INTENT",
"RESET_CONFIG": "true",
"PARAM_LIST": {
"intent_output_enabled": "true",
"intent_action": "com.zebra.ionicdemo.ACTION",
"intent_delivery": "2" // Broadcast
}
}
};
(window).plugins.intentShim.sendBroadcast({
action: 'com.symbol.datawedge.api.ACTION',
extras: {
"com.symbol.datawedge.api.SET_CONFIG": profileConfig2,
"SEND_RESULT": this.requestResultCodes
}
},
function () { }, // Success in sending the intent, not success of DW to process the intent.
function () { } // Failure in sending the intent, not failure of DW to process the intent.
);
Putting this all together, you now have an application that will receive barcodes from the default device scanner without having to perform any special scanner control logic or write any native code.
So far, we have shown the DataWedge API being used to create a profile and configure the scanner. There are far more APIs available. For example, if you wanted to switch from the default imager scanner to a connected Bluetooth scanner, you could use the SWITCH_SCANNER API (DW 6.5+)
(window).plugins.intentShim.sendBroadcast({
action: 'com.symbol.datawedge.api.ACTION',
extras: {
"com.symbol.datawedge.api.SWITCH_SCANNER": "" + 3 // Assume 3 is the index of the BT scanner returned from a call to ENUMERATE
}
},
function () { }, // Success in sending the intent, not success of DW to process the intent.
function () { } // Failure in sending the intent, not failure of DW to process the intent.
);
Demo Application
I have put together a basic demo application at https://github.com/Zebra/ZebraIonicDemo that enables you to scan barcodes, change the scanner, and configure the decoders in use.
The application will work on any Zebra mobile computer running Android that supports the DataWedge service. Depending on the version of DataWedge running on your device, some features might not be available to you, but all devices support scanning and capturing barcode data at a minimum. Please see the demo readme file for more information and setup.
Building and Running the Demo Application
- cd ZebraIonicDemo
- npm install
- Connect Zebra device to adb
ionic cordova run android --device
Please raise any issues with the demo app in GitHub
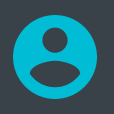
Anonymous (not verified)
15 Replies
Hi,
is there any possibility to use SimulScan (OCR documents) in Ionic app?
Hi, thank you for your answer, but version of my DataWedge is 6.6.50 (Zebra TC56:7.1.2). Is there any possibility to update DataWedge on this device?
Help I need to print on the Zebra Imz320 printer, is there an example with DataWedge?
Hi, you cannot use DataWedge for printing I'm afraid, for more information on our Printing SDKs please see the following techdocs link: <a href="http://techdocs.zebra.com/link-os/2-14/">Available Product Documentation - Zebra Technologies Techdocs</a> or the product support page: <a href="https://www.zebra.com/us/en/support-downloads/printers/mobile/imz320.ht… Mobile Printer Support & Downloads | Zebra</a>
Hi Darryn ,
thanks very much, i have three accessory :1、testApp.apk is my test apk 2、I recorded operation video 3、my project
my project has two page ,The LoginPage and scanPage , the scanPage is copied from yours.i first came into my scanPage ,it worked well ,when i exit to my LoginPage then back to scanPage ,i press the scan key ,it not work , when i press scan key several times, it can work ,when i exit to LoginPage again, then back to scanPage ,the scan key cannot work.
Looking forward to your reply
Hi, did you send your project? I do not see it - perhaps it was blocked by the email filter.
Hi, you need to configure the DataWedge profile to specify the correct associated application (step 5 at this link <a href="http://techdocs.zebra.com/datawedge/7-0/guide/createprofile/#createanew… Profiles - Zebra Technologies TechDocs</a> ). I also emailed you a video for context but it was 17MB so it might not get through. Let me know if you don't receive it and I'll share it another way.
can you help me?
your demo is shown at the root, i have a login page,directory page ,then my scanpage,when i first goto scanpage then press scan key ,it ok, but when i go back to directory page ,then enter scan page again ,i pressscan key ,but it not work? is events.subscribe go wrong?Looking forward to your reply
Hi Darryn ,
I add your barcode.ts into my project, and the main code (subscribe events) into my ts.file ,first time i get scan events ,but when i go to other page then i go back to the main page ,i cannot get scan events, can i send my ionic project to you ?
looking forward to a reply!
Hi, it should work although I have never tried it. SimulScan support was added to DataWedge 6.8 - you should be able to change the SET_CONFIG call to use the SIMULSCAN device_id (<a href="http://techdocs.zebra.com/datawedge/6-8/guide/api/setconfig/">Set Config - Zebra Technologies TechDocs</a> ). There was also another API introduced in DataWedge 6.8, Switch Params, <a href="http://techdocs.zebra.com/datawedge/6-8/guide/api/switchsimulscanparams… SimulScan Params - Zebra Technologies TechDocs</a> to specify the template etc.
Hi Darryn
Thanks for your email, i confirm my DataWedge profile has No problem, i sent you a video that shows what happening about Last Api message , i guess there's a problem , but i don't know what happened?
Thanks
huahua
Hi, the version of DataWedge can only be updated by upgrading the device OS. This can be done via a LifeGuard patch: <a href="https://www.zebra.com/gb/en/products/software/mobile-computers/lifeguar… | Mobile Computer Software | Zebra</a> .
You can download updates for TC56 from <a href="https://www.zebra.com/us/en/support-downloads/software/operating-system… Operating System For GMS Devices Support and Downloads | Zebra</a> or <a href="https://www.zebra.com/us/en/support-downloads/software/operating-system…; . It appears that the latest LG update, 08, also includes DataWedge 6.8
I need to print from Ionic 3 on the Zebra iMZ320 printer, is that possible? Is there an example? Thank you
Hi hua hua,
The demo only uses Ionic events (<a href="https://ionicframework.com/docs/api/util/Events/">https://ionicframewor…; ) to communicate between a barcode "provider" and the main application - if you are not getting scan events the first thing to debug would be whether the intents are being received in your broadcast receiver (corresponding to this line in the sample app: <a href="https://github.com/Zebra/ZebraIonicDemo/blob/master/src/providers/barco… at master · Zebra/ZebraIonicDemo · GitHub</a> )